Migrating from 4D
4D is a database application development environment. It is often used to create specialized in-house database applications. If you have an 4D application and are running into limitations, Xojo is a great choice to take your application to the next level.
Similarities
Like 4D, Xojo is an IDE that includes a layout editor, code editor, debugger, compiler, object-oriented programming language and more.
Like 4D, Xojo provides a library of user interface controls for desktop, mobile and web as well as an extensive framework of functions that abstracts you from the details of the underlying operating systems.
Differences
Unlike 4D, Xojo layouts (windows, webpages and mobile screens) are not tied to database tables. They are independent objects. Because of this, data from rows are not loaded automatically into the user interface controls. While you can write code easily enough to do this, a better option is to use DBKit which is a free add-on for Xojo that can load data into controls without you having to write code.
Unlike 4D, Xojo is designed to work equally well with MySQL, PostgreSQL, ODBC and SQLite. In fact, nearly all the code you write to access these databases will be identical regardless of which database engine you choose to use.
Unlike 4D, there is no per end-user licensing fee or runtime license of any kind. The purchase of your Xojo developer license allows you to deploy as many applications to as many end users as you like.
Unlike 4D, Xojo uses SQL as its exclusive database query language. However, it provides an extensive database API that abstracts you from SQL with the sole exception of queries.
Migrating
The eight steps for migration are as follows:
Make a list of any functions upon which your application depends and make sure that those functions are available in Xojo, in a Xojo add-on or can be replaced by you via some other means.
Recreate your database schema in the database engine of your choice.
Export the data from your existing 4D database and then import into your new database file. There are database tools such as TablePlus that make this easy. If the data is likely to change before you are finished migrating your application, you will of course have to do this again but it's a good idea to have very good sample data while recreating your project in Xojo.
Recreate your layouts in Xojo. This may seem like a huge job (and if you have a lot of layouts, it likely will be) but keep in mind that you spent a long time deciding how the layouts should look. Recreating them doesn't require redesigning them and as a result the process should go much faster.
Recreate your logic in Xojo. If you've created classes in 4D you should be able to create similar classes in Xojo. Make sure to start by going through the Xojo QuickStart and Tutorials. Read about DBKit and the Database classes such as Database, RowSet and more. If you need help with understanding how things work, check the documentation, example projects (which can be found right in the Xojo IDE by choosing File > New Project then clicking on the Examples tab), ask on our user forum or contact us directly.
You may be able to use the built-in Xojo report editor for some of your reports. Otherwise, printing can be done via the Graphics class. Anything you can draw via the Graphics class can be displayed on the screen or sent to the printer.
Initial testing can be done on your development machine. You'll want to make sure to test on all platforms that you intend to support. Xojo's Remote Debugger makes it easy to test on other operating systems.
Ship!
Forms/Layouts
Layouts in Xojo work similarly to 4D. Unlike 4D they do not have sections. Creating them is essentially the same as 4D in that you drag and drop to add controls and then use the Inspector pane to configure them. If you need to display a list of rows, the best solution is to use a DesktopListBox, WebListBox or iOSMobileTable or AndroidMobileTable depending upon the type of project you are creating. If your rows are taller and more complex than a ListBox allows, there are other options such as using Containers (DesktopContainer, MobileContainer and WebContainer as well as HTMLViewers (DesktopHTMLViewer, MobileHTMLViewer, WebHTMLViewer).
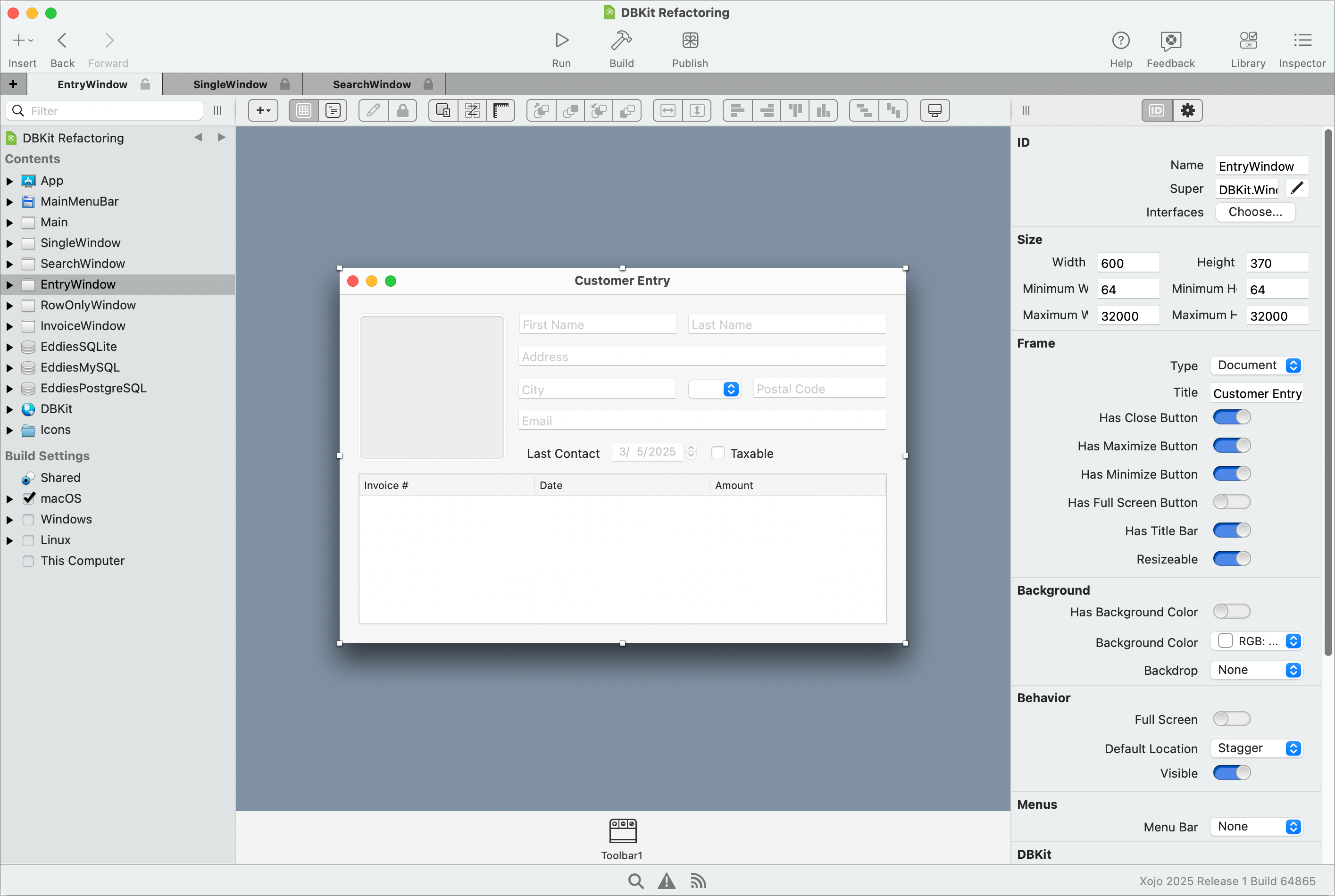
4D to Xojo Project Converter
This app can convert 4D forms into Xojo desktop windows. It's open source. You can download it here.
Source code
Xojo is event-driven and includes an object-oriented language that is strongly-typed. Desktop, Mobile (Android and iOS) and Web projects will have an Application class (DesktopApplication, MobileApplication, WebApplication) that has an Opening event which where your application's code executes first as your app is launched and a Closing event that is the last code that executes when the application is shutting down. This Opening and Closing event pairing also exists for layouts, controls and some other classes.
In Xojo the user interface class names, property names, method names and more are generally the same regardless of project type. There are of course some differences as there are features that are unique to a particular project type.
Most of the classes that are not user interface-related are supported for all project types and operating systems. This makes it easy to use them across operating systems and even project types.
Xojo's backend compiler is LLVM which is an optimizing compiler. Apple's XCode and many other tools use LLVM.
Example code
This is example code connects to a local SQLite database, finds a row and then updates it.
Var db As New SQLiteDatabase
db.DatabaseFile = New FolderItem("CompanyData.sqlite")
Try
db.Connect
Var rows As RowSet
rows = db.SelectSQL("SELECT * FROM Employees WHERE ID=134560")
rows.EditRow
rows.Column("YearsOfService").IntegerValue = 5
rows.SaveRow
db.CommitTransaction
Catch error As DatabaseException
MessageBox("Error: " + error.Message)
db.RollbackTransaction
End Try
Xojo uses the concept of an exception to deal with error handling. The assumption is that no errors will occur and that should one occur, that is an exception. Code is placed in a Try Catch statement. Should any database error occur on any line inside the statement, the Catch portion will be executed. In most cases, an error is not recoverable and the user will have to start the operation again.
In a real application, the db variable would likely be a property in a global location such as the App object that every project has or in a web application the Session class.
If this code was instead connecting to a database server, the only change would be that db variable would be set to a difference database class and the additional info need (such as the IP address of the server) would be provided:
Var db As New PostgreSQLDatabase
db.Host = "192.168.1.172"
db.Port = 5432
db.DatabaseName = "CompanyData"
db.AppName = "MyApp"
db.Username = "Charlie"
db.Password = "mashie"
Protecting Your Database
Xojo uses the concept of Prepared Statements to prevent SQL-Injection attacks. Anytime your code is going to perform a query with data entered by the user, make sure you use Xojo's SelectSQL syntax that allows you provide those values as separate parameters allowing the database engine to make sure they don't have any SQL commands embedded within them.
Code editor
The Code Editor includes syntax highlighting, autocomplete, syntax help, refactoring tools, documentation access and more.
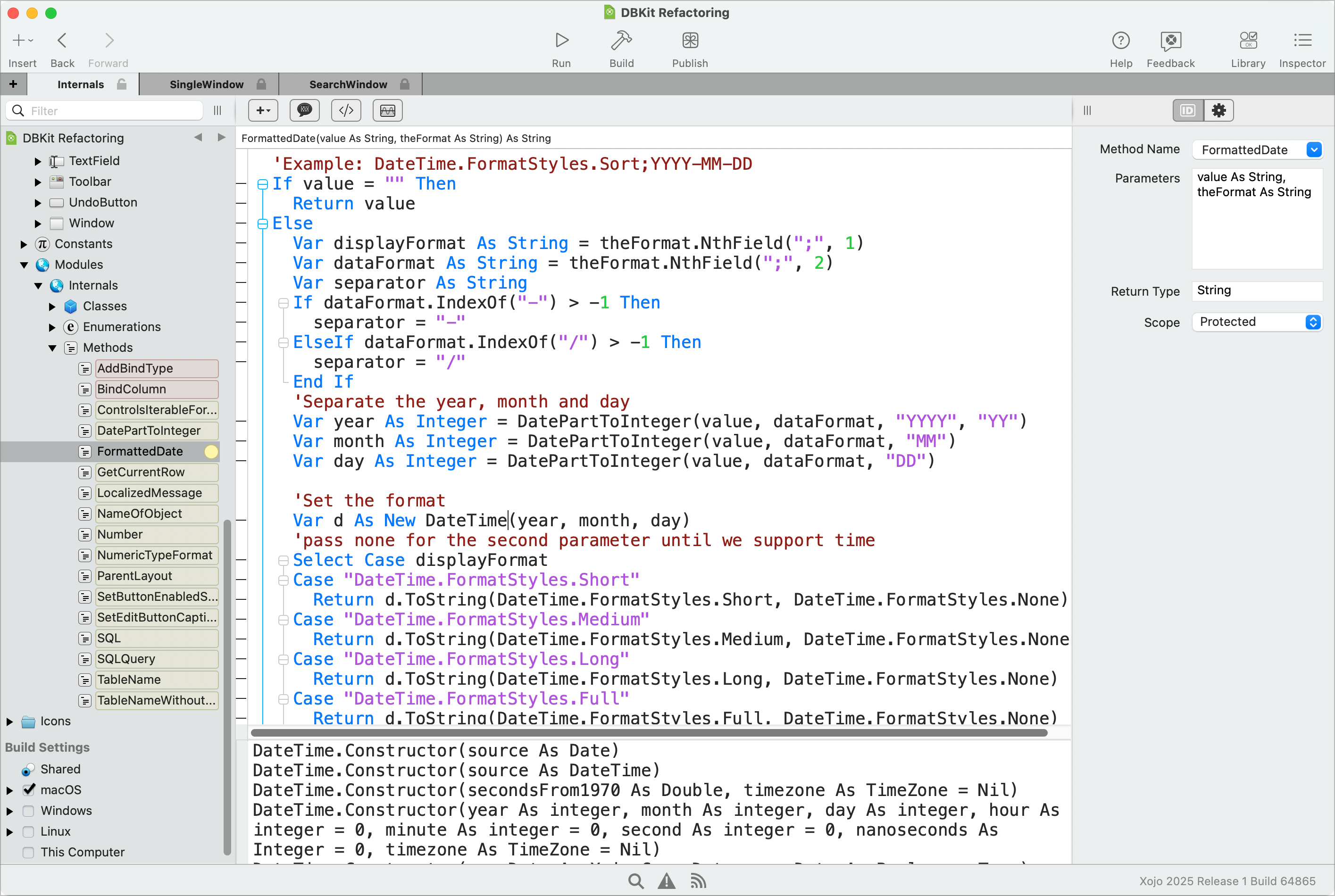
Debugger
When you run your project from the Xojo IDE it is compiled to a binary application with debug code so that it can communicate with the Xojo IDE's Debugger. Desktop applications are launched, web apps open in your browser and mobile apps can be run either in the simulator or on a mobile device. With the Remote Debugger, Xojo can send your compiled app to a remote computer or local virtual machine to run while you debug from the Xojo IDE on your computer.
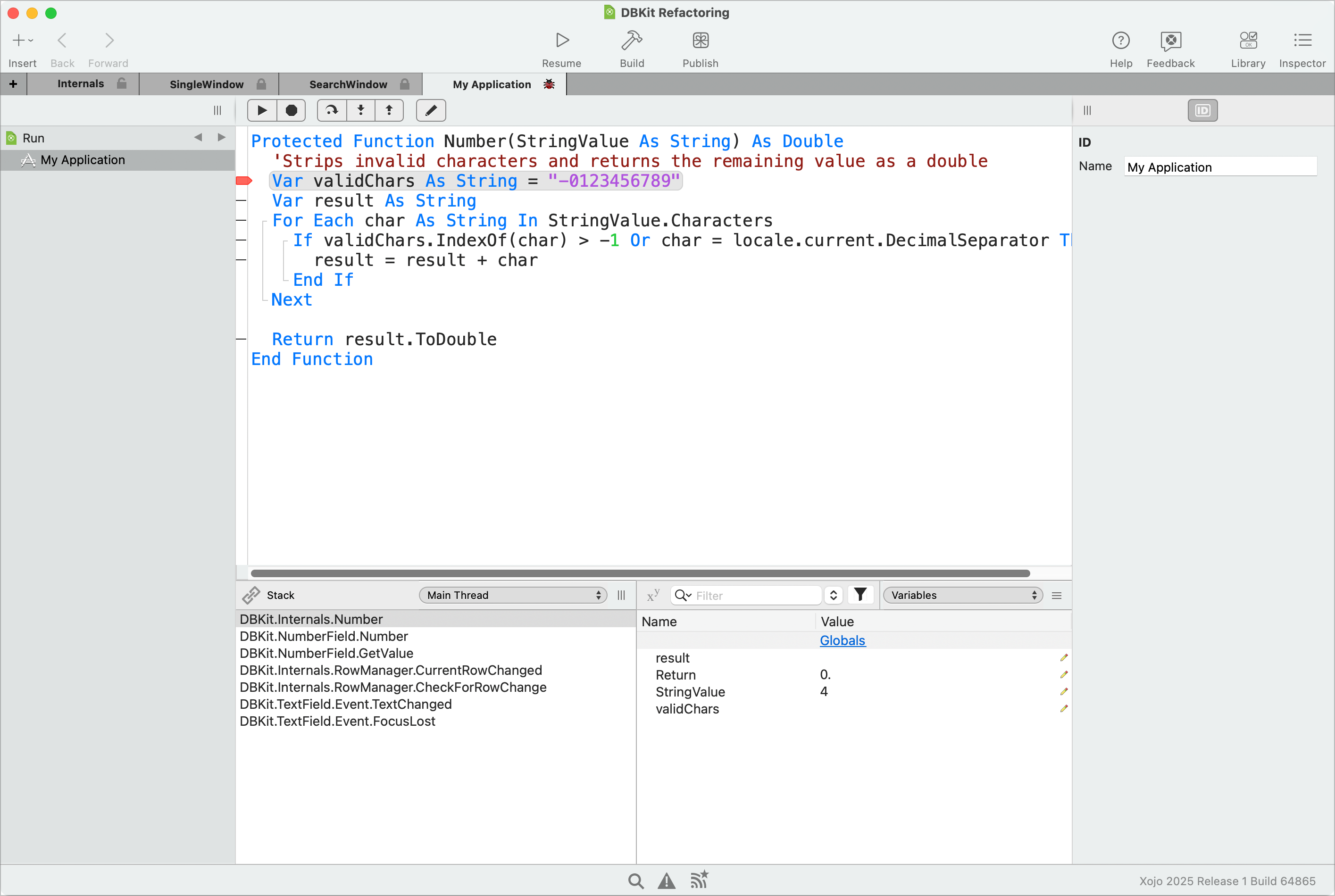
Version control
By default, Xojo projects are saved in a binary format. However, for use with version control you can choose Xojo Project format which is a text-based format where each project item is a separate text file.
Deploying your app
With Xojo you can build desktop applications for Linux, MacOS and Windows. They can be deployed in any way you deploy your desktop applications today. MacOS desktop applications can additionally be deployed via the Mac App Store directly from the Xojo IDE. You can build web applications that can be deployed on any server that will allow you to run a console application or quite easily via Xojo Cloud. Mobile applications for iOS and be deployed directly to the iOS App Store right from the Xojo IDE. They can also be deployed through Apple's Enterprise deployment offering. Android applications can be submitted to the Google Play store, other stores or directly to the device.
Mobile operating systems do not allow applications to keep synchronous connections open very long as they use up too much energy and run down the battery. To avoid this, you will need to use an asynchronous connection. It is recommended that you do this by creating a Xojo web application that acts as the middleware between your mobile app and your database. You can then use Xojo's URLConnection class to communicate with it asynchronously.