Understanding the Message Box
A MessageBox displays a prompt for the user. Although it is a user interface control, it does not appear on the Layout so when you drag it to your Layout, it is added to the Shelf.
Unlike with desktop a dialog (but similar to a web dialog), the Mobile Message Box is not modal. Your code does not stop executing when the Message Box is displayed. If you have code you want to run after the Message Box is closed, then put it in the Pressed event.

Below are common events, properties and methods. Refer to MobileMessageBox for the complete list.
Events
Pressed - This event is called when one of the buttons on the Message Box is tapped, with a parameter containing the index of the button that was tapped.
Properties
Methods
Usage
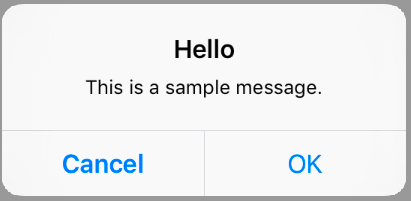
The easiest way to use a Message Box is to drag it from the Library onto the Layout. The Inspector for a Message Box can be used to change the Message, Title, Left Button and Right Button properties. If you need more than two buttons, you can add them using code. When there are more than two buttons, they display vertically. This example adds three buttons to a Message Box that has been added to the Screen:
Var buttons() As String
buttons.Add("Yes")
buttons.Add("No")
buttons.Add("Maybe")
HelloMessage.Buttons = buttons
HelloMessage.Show
It is important to understand that your code does not pause at the "Show" call to wait for a button to be tapped. A Message Box is asynchronous, which means it displays immediately and your code continues running to the end of the method or event.
Note
Calling the Show method does not pause your code until a button is tapped.
To determine when a button was tapped, you must add the Pressed event handler to the Message Box. This event is called with a parameter containing the index of the button that was tapped. This code displays the index for the tapped button:
Label1.Text = "You selected button " + buttonIndex.ToString
More advanced techniques
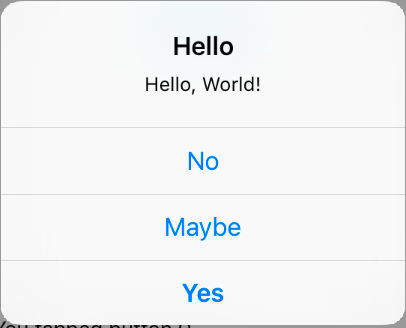
You can also add a Message Box entirely in code, but you'll need to provide a method (on the View) to handle the Pressed event (using AddHandler). To add a simple Yes/No Message Box, start by adding a property to the Layout:
SampleMessageBox As MobileMessageBox
In the code that displays the Message Box, create it and tell it to call a method when the Pressed is called. You might do this on a Button:
SampleMessageBox = New MobileMessageBox
SampleMessageBox.Title = "Hello"
SampleMessageBox.Message = "Hello, World!"
Var buttons() As String
buttons.Add("OK")
SampleMessageBox.Buttons = buttons
AddHandler SampleMessageBox.Pressed, AddressOf SampleButtonPressed
SampleMessageBox.Show
Now add the method to the Screen to perform an action and remove the handler:
Private Sub SampleButtonPressed(sender As MobileMessageBox, index As Integer)
OutputLabel.Text = "Button tapped."
RemoveHandler SampleMessageBox.ButtonPressed, AddressOf SampleButtonPressed
End Sub
See also
MobileMessageBox class; Creating Dialog Boxes topic