Data types
Xojo is a strongly-typed programming language. This means that you must specify the type of every variable you create. Strongly-typed programming languages are safer to use because the compiler can inform you of programming mistakes in variable usage before they make it into your app.
Xojo has several built-in data types (called intrinsic types) which fall into these categories: Boolean, String, Numbers (Integer, Double and others) and Color.
In addition, DateTime is a commonly used class that is often considered a general type.
Integers, Doubles and Colors are also called value types because they contain the actual value you assign to them. Strings, arrays and any class types are called reference types because they actually contain a pointer to the value, but you don't have to specifically worry about that now.
Boolean
Boolean is one of the simplest data types. It can only contain one of two values: True or False (the default). This is how you declare a Boolean variable:
Var b As Boolean
The special keywords True and False used to change the value of a Boolean variable. If you want to declare a Boolean variable and assign it to True, you can do so in one step:
Var b As Boolean = True
Boolean variables can also be assigned the result of a Boolean expression. A Boolean expression is an expression that evaluates to True or False. For example, this code sets a variable to True or False depending on whether an Integer value is greater than 5:
Var i As Integer = 10
Var b As Boolean
b = (i > 5) ' b gets set to True
Boolean values are often used with controls. For example, code such as this tests if a CheckBox has been checked:
If AutoSaveCheckBox.Value = True Then
' your code here
End If
A Boolean value is its own expression so you could simplify the above by leaving off the comparison:
If AutoSaveCheckBox.Value Then
' your code
End If
Some developers prefer to keep the "= True" part because it is more explicit, but that is a preference that varies from developer to developer.
Boolean expressions
Boolean expressions deal only with the values True and False. True and False are values that Xojo can manipulate, just as it does numbers and strings. There are two types of Boolean expressions:
Simple: Boolean expressions state something about a value in the program, generally using operators like = (equals), <> (not equals), <= (less than or equal), or >= (greater than or equal). Sometimes a property or something else in the program will actually just be a Boolean value. The toggle switches you see in the Inspector are good examples, such as the Visible property of a control. For example, Label1.Visible evaluate to True when the label's Visible switch is set to ON; and it evaluates to False when the switch is set to OFF.
Compound: Boolean expressions that are made out of other Boolean expressions (which can themselves be either simple or compound), using Boolean operators Not, And or Or.
As with other types of expressions, you will often need to use parentheses in Boolean expressions to make it clear in what order operations should be carried out. Also, as with other types of expressions, Xojo works out the final value from the inside out.
Example expressions
Consider this expression:
(Source1.Text.Val = 0 And Source1.Text <> "0") Or (Source2.Text.Val = 0 And Source2.Text <> "0")
A compound expression such as this is evaluated according to the left-to-right rule. The two branches of the Or expression are evaluated separately prior to Or-ing them.
If a compound expression is joined by And, then any sub-expression that evaluates to False ends the process of evaluating any subsequent sub-expressions within that compound expression. This is because one False sub-expression means that the entire compound And expression must also be False.
An expression like this can be represented as a syntax tree:
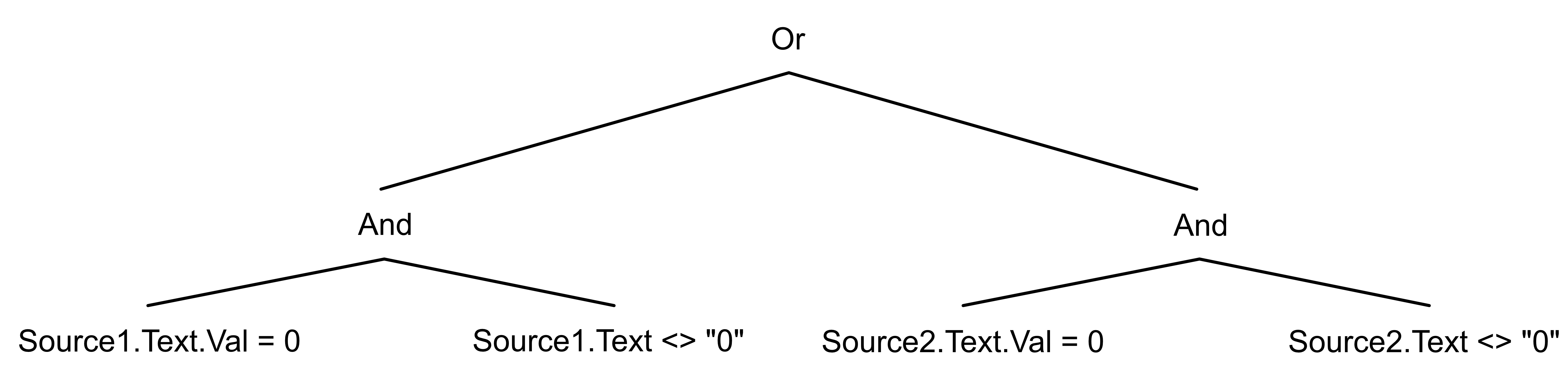
The expression is evaluated from the bottom of the tree up. Each level of the tree is evaluated left to right.
In this example, the first step is to evaluate the left branch of the Or expression. It is a compound expression of its own, as its branches use And. It starts with
Source1.Text.Val = 0
If this expression returns True then it evaluates:
Source1.Text <> "0"
Lastly, if
Source1.Text.Val = 0
is False, there is enough information to evaluate the And so False is returned to the next higher level without evaluating the second expression. Since the two compound expressions are joined by Or, it needs to evaluate the right compound expression at the base of the tree even if the left compound expression evaluates to False. If the left branch is False, but the right branch is True, then the complete compound expression is True.
The same procedure is followed in evaluating the right branch of the compound Or statement.
Strings
A String is any series of characters. Use the String data type to store a series of characters.
The maximum size of a String is approximately 4GB. To declare a variable for containing text:
Var firstName As String
The default value of a String is the empty String, often written as "":
Var firstName As String
If firstName.IsEmpty Then
' Do something if string is empty
End If
You can also directly assign text to the variable as part of its declaration:
Var t As String = "Hello, World!"
Basically, any type of characters can be stored as a String, such as: "Lucas", "12/30/2015", "42.15".
Yes, even though those last two values look like a date and a number, they are actually Strings because they are a series of characters. If you want to treat them as if they are an actual date or a number then you need to use a specific data type for that.
Combining text
You can combine multiple text values together by using the addition (+) operator. This concatenates the two values together. For example:
Var name As String = "Lucas" ' no space at end
Var age As String = "11"
Var combined As String = name + age ' result is "Lucas11"
Remember, that text values combined in this manner are only ever concatenated, regardless of what value they contain. For example, even if it looks like the string contains numbers, adding them will not calculate their sum:
Var value1 As String = "42"
Var value2 As String = "10"
Var value3 As String = value1 + value2 ' result is "4210", not "52"
String methods
String has many methods that can be used to manage its contents. For example, you may want to check if a String variable contains another String value or you may want to remove whitespace from it.
To learn more about the methods that are available with String variables, refer to the String data type.
Encodings
In order to store text outside of your apps (in files or databases, for example), the text needs to be encoded. When text is encoded, it is converted to a series of bytes that can be processed by other apps and systems. If you are loading text from these outside sources into your app, then you'll also need to know the encoding so you can set it using the DefineEncoding method.
The most common encoding for Unicode text is UTF-8, but if you may run across other encodings, such as ASCII, Win-Latin, MacRoman, etc.
Refer to the Encodings section for more information.
Numbers
The Xojo programming language has separate data types depending on the type of number you need. The Integer type is used for whole numbers (positive or negative and 0). If you need a number that contains a decimal, you can use either Double or Currency.
Because these data types contain actual numbers, you can perform mathematical computations on them.
Integer
An Integer contains whole numbers and is declared like this (default value is 0):
Var value As Integer
You can assign it a value when you declare it:
Var value As Integer = 42
If you try to assign a value with a decimal to an Integer, the decimal value is truncated (not rounded) before it is assigned. For example:
Var value As Integer
value = 42.65 ' value will contain 42
You can perform mathematical calculations on integers:
Var i1 As Integer = 42
Var i2 As Integer = 8
Var sum As Integer
sum = i1 + i2 ' sum = 50
Var product As Integer
product = i1 * i2 ' product = 336
Var div As Integer
div = i1 / i2 ' div = 5 (truncated)
Double
A Double is a number that can contain a floating-point decimal value. In other languages, Double may be referred to as a double precision real number. Because Doubles are numbers, you can perform mathematical calculations on them.
The default value of a Double is 0.0:
Var value As Double ' value is 0.0
You can also assign a value when you declare it:
Var value As Double = 3.14
You should avoid using Double to store monetary values. As an IEEE floating-point number, there are some decimal values that cannot be represented as a Double and this could cause rounding issues. In these situations, use Currency instead.
For example, consider this code:
Var d1 As Double = 5.1
Var d2 As Double = d1 * 100
Var d3 As Double = Round(d1 * 100)
The value 5.1 cannot be stored directly in a Double (it's actually more like 5.0999999999999996). When you multiple that actually value by 100 you end up with 509.9999999999999432 (this is d2) when you are really expecting a value of 510. In this case you want to use the Round method to get the value you want (d3).
Note
Learn more about how floating point values are stored by reading A Floating Point Values Primer.
Currency
Currency is a fixed-point number format that holds approximately 15 digits to the left of the decimal point and 4 digits to the right. It is always accurate to four decimal places. Currency is useful for calculations involving money and for calculations where accuracy is very important. It is compatible with the Currency data type offered in some versions of Visual Basic.
The default value of a Currency variable is 0.0.
Here is are some declarations:
Var value As Currency
Var amount As Currency = 42.56
Mathematical computations
Performing mathematical calculations is a very common task in programming, and all of the common mathematical operations are supported for the number data types.
This is a list of mathematical operators:
Operation |
Operator |
Example |
---|---|---|
Addition |
2 + 3 = 5 |
|
Subtraction |
3 - 2 = 1 |
|
Multiplication |
3 * 2 = 6 |
|
Floating Point Division |
6 / 4 = 1.5 |
|
Integer Division |
6 \ 4 = 1 |
|
Modulo |
6 Mod 3 = 0, 6 Mod 4 = 2 |
|
Exponentiation |
2 ^ 3 = 8 |
There are also many built-in mathematical operators and functions that are covered in detail in the Documentation.
Expressions support standard mathematical precedence. This means that equations surrounded by parentheses are handled first. The expression is evaluated beginning with the set of parentheses that is embedded inside the most outer sets of parentheses. Next, exponentiation is performed, followed by any multiplication or division from left to right. Finally any addition or subtraction is performed. As an example, these three expressions return different results because of the placement of parentheses.
Expression |
Result |
---|---|
2 + 3 * (5 + 3) |
26 |
(2 + 3) * (5 + 3) |
40 |
2 + (3 * 5) + 3 |
20 |
For more information, refer to the Operator Precedence topic.
Converting between numbers
You can convert between numbers without any special commands, but you have to keep in mind that the numbers may be rounded or truncated. For example, converting a Double to an Integer does not round the value, but instead truncates it to the integer part:
Var d As Double = 4.6
Var i As Integer = d ' i = 4
Use the Round method to round a value.
Var d As Double = 4.6
Var i As Integer = Round(d) ' i = 5
You can also directly assign an Integer to a Double:
Var i As Integer = 42
Var d As Double = i ' d = 42.0
For math that has values that are both Doubles and Integers, then a Double is used to calculate the value.
For example, consider this code:
Var result As Integer
result = 5.1 * 8
In the above case, the expression is calculated as 5.1 * 8, which is 40.8 and then that is assigned to the Integer variable for result, which then truncates it to 40.
If you wanted to Round that to 41, then you would use the Round method like this:
Var result As Integer
result = Round(5.1 * 8) ' result = 41
Color
Color is a data type that stores the value of a color. A Color “value” actually consists of three numeric values that can be set using any of the three popular color models, Red-Green-Blue, Hue-Saturation-Value, or Cyan-Magenta-Yellow. Each RGB value is stored as a byte. That means that each number can take on 256 possible values.
A common way to create a specific color is to use the RGB method. This creates a color variable containing a blue-gray color:
Var c As Color = Color.RGB(124, 124, 163)
You can also assign color values using some of the Color constants or by directly specifying hex values using the &c operator:
Var blueColor As Color = Color.Blue
Var greenColor As Color = &c00ff00
DateTime
DateTime is a class and not an intrinsic data type. But it is commonly used and worth mentioning here. Because it is a class, you have to create an instance of it before you can use it. This is also means it is a reference type.
Because DateTime is a class, you use the New operator to create a new date like this:
Var d As New DateTime(2017, 4, 15)
The best way to compare dates is to use the SecondsFrom1970 property like this:
Var d1 As New DateTime(2017, 4, 15)
Var d2 As New DateTime(2018, 4, 15)
If d1.SecondsFrom1970 > d2.SecondsFrom1970 Then
' do something
Else
' do something
End If
Calculating intervals between dates
The Now method which gives you the current date and time:
Var d As DateTime = DateTime.Now
You can also create a specific date by providing the values like this:
Var d As New DateTime(2019, 8, 20, TimeZone.Current) ' Aug 20, 2019
Once you have a DateTime, you cannot modifiy it. You can however subtract dates to get a DateInterval of the time between the two dates or you can add a DateInterval to a date to get a new DateTime.
This code gets a date two months before today:
Var twoMonths As New DateInterval
twoMonths.Months = 2 ' 2 month interval
' Get date two months before today
Var past As DateTime = DateTime.Now - twoMonths
This calculate the interval until January 1, 2030:
Var d2 As New DateTime(2030, 1, 1, TimeZone.Current)
Var interval As DateInterval
interval = d2 - DateTime.Now
' Check the Years, Months, Days, etc. properties
To display dates you use the ToString method. This method lets you specify the format you want for the date part and the time part. You can also hide one of those parts. For example, This code creates a date for 31-Oct-2017 at 11:00am and then converts it to text in several different ways:
Var myDate As DateTime(2017, 10, 31, 11, 00, 00, TimeZone.Current)
' Get just the date part
Var dateValue As String = myDate.ToString(Locale.Current, _
DateTime.FormatStyles.Short, DateTime.FormatStyle.None)
' Get just the time part
Var dateValue As String = myDate.ToString(Locale.Current, _
DateTime.FormatStyles.None, DateTime.FormatStyles.Short)
' Get the full date and time
Var dateValue As String = myDate.ToString(Locale.Current, _
DateTime.FormatStyles.Short, DateTime.FormatStyles.Short)
To convert a string that contains a date back to a DateTime instance use the FromString method. Since the format for dates varies greatly by locale when storing dates as strings you should always use the standard SQLDateTime format (YYYY-MM-DD HH:MM:SS).
You can get a date in SQL format using the SQLDate or SQLDateTime methods:
Var sqlDate As String = DateTime.Now.SQLDate
A date in that format can be converted back to a DateTime instance using the FromString method like this:
Var SQLDateTime As String = "2015-08-01 11:00"
Var myDate1 As DateTime = DateTime.FromString(SQLDateTime)
Typeless variables
There may be times where you need a variable that can contain the value of any other variable, regardless of its type. The Variant data type is used for this purpose.
Variant
The Variant type can contain a value of any type and has the ability to implicitly convert its values to other data types. Use this with caution as it can result in hard-to-find bugs in your code when you run into unintended behavior from type conversions.
Converting between data types
There may be times when you need to change a value from one data type to another. This is usually because you want to use the value with something that is designed to work with a different data type. For example, you might want to include a number in a Label's Value property, but the Value property's data type is String, not a number. Consequently, if you try to assign a number to the Value property, you will get a type mismatch error when you try to run your project.

Instead, you need to convert the number to the appropriate type. Most commonly, you will need to convert from numbers to strings and from strings to numbers. You can do this using the ToString and FromString methods on the data types.
For example, to convert an Integer to a String:
Var i As Integer = 42
Var num As String = "The number is " + i.ToString
To convert a String to an Integer:
Var value As Integer
value = Integer.FromString("42") ' value = 42
You may also want to display a DateTime as a string. This code display the current date and time:
Var d As DateTime = DateTime.Now
Label1.Text = d.ToString