Properties
A property is a value of a class or module. You can think of it as a variable that belongs to a class or module. Most of the built-in classes have properties. For example, the DateTime class has a property to get the Year.
For more about using properties with Modules, refer to the Modules topic. For more about properties when used with classes, refer to the Class Properties, Methods and Events topic.
There are two types of properties you can add: a standard property and a computed property.
Property
Properties can be added to project items, including classes (including Windows, Web Pages, iOS Views) and modules. There are several way to add a property:
Insert button on the main toolbar
Add button on the Code Editor toolbar
Insert > Property from the menu
The contextual menu
Keyboard shortcut (⌥ ⌘ P on macOS or Ctrl Shift P on Windows and Linux)
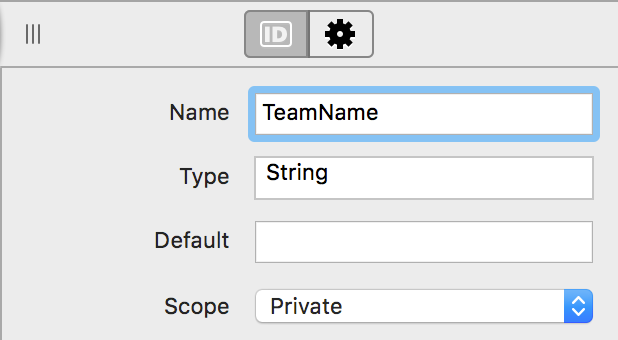
To quickly create a property, you can enter both its name and type on one line in the Name field like this: PropertyName As DataType. When you leave the field, the type will be set in the Type field.
Any of these steps adds the property, displays a blank editor (where you can type notes) and displays the Inspector where you can set the values for the property. These are the values you can change:
Name
The name of the property. Just like variables, properties are given names to describe them and the same naming rules apply. To create a property that is an array, add parenthesis after the name just as you would when creating an array variable.
Type
The Type can be any valid data type, including classes.
Default
The default value for the type. For example, for an Integer property you can specify 42 to have its default value be 42. You can only set default types for the intrinsic properties (Integer, Double, String, etc.) and not for object types.
Scope
Scope indicates what parts of your code can access the property. Choices are Global, Public, Protected and Private.
Global properties (used with Modules) can be used anywhere in your code with no restrictions.
Public properties (used with Classes) can be called from anywhere in your code with no restrictions.
Protected properties when used in classes can be used only within the class itself or within a subclass. When used with modules, the property can be used only in conjunction with the module name.
Private properties can only be called by the module or class that contains the property.
Note
Scope is indicated in the Navigator via the background color of the item. An item that is public will have no background color. An item that is Protected has a yellow background. An item that is Private will has a red background.
The property signature (its name, and type) also appears at the top of the Editor for reference.
Properties are referenced by their name when used within the module or class that owns them. This is how you would reference a TeamName property in your code:
TeamName = "Flying Squirrels"
For usage outside of the class or module that owns them, they are often referenced differently (usually with the class or module name as a prefix). Refer to the Modules and Classes topics for specifics.
Computed property
A computed property is a property that does not store its value but instead calculates it each time it is accessed. This means a computed property does not store an actual value. Instead it uses a standard property to store a value or contains code to calculate a value.
There are several ways to add a computed property:
Insert button on the main toolbar
Add button on the Code Editor toolbar
Insert > Computed Property from the menu
The contextual menu
Like with a property, you have to specify the values for the computed property, which are:
Name
The name of the property. Just like variables, properties are given names to describe them and the same naming rules apply.
Type
The Type can be any valid data type, including classes.
Scope
Scope indicates what parts of your code can call the method. Choices are Global, Public, Protected and Private.
Global computed properties can be used anywhere in your code with no restrictions. These are available only with Modules.
Public computed properties can be called from anywhere in your code with no restrictions. These are available only with Classes.
Protected computed properties have some restrictions, which vary depending on where the method is located (class or module).
Private computed properties can only be called by the module or class that contains the method.
The property signature (its name, and type) also appears at the top of the Editor for reference.
A computed property has two additional parts to it: a Getter and a Setter. A computed property can be expanded in the Navigator to display the Get and Set sections below the property name. You add code to the Get section to get or calculate a value for the property. You add code to the Set section to set a value for the property. If you do not include code in Set then the property becomes a read-only property. You can also leave out code in the Get section to make the property write-only, but that is less common. In the Navigator, the Get and Set sections appear as bold when they have code.
An example of a computed property might be a TotalPrice property of a Product class. You could implement TotalPrice with this code in Get:
Return Price * Quantity
If your computed property needs to retain a value, often you would create a matching private property to do so (usually prefixed with an “m”). For a computed property called "TeamName As String", this means you might do something like this:
Get:
Return mTeamName
Set:
mTeamName = Value
The above computed property is referring to a companion property:
mTeamName As String
Code can access the computed property by its name:
Var tName As String = TeamName
For usage outside of the class or module that owns the computed property, they are often referenced differently (usually with the class or module name as a prefix). Refer to the Modules and Classes sections for specifics.
Although the behavior is different, using a computed property in this manner is not much different than a regular property. There is one notable benefit: you can set a breakpoint in the Set section so you can track when the property value changes.
Computed properties appear in the debugger, but it is important to note that the debugger will call the "Get" section of code in order to get the value to display. This may have unintended side-effects depending on how your code works.
Note
Computed properties cannot be array types.