Methods
Method
Now that you understand the various coding concepts, it is time to learn more about the place where you write you code: methods.
Methods are the building blocks of your application. The code you write most often exists in a method. A method is one or more instructions that are performed to accomplish a specific task; an action of some sort.
Xojo has many built-in methods. Some are global such as the Quit method that causes your application to quit. Most framework classes have built-in methods. For example, the DesktopListBox class has a method called AddRow for adding rows to it (as the name implies). And you can of course create your own methods.
Creating your own methods
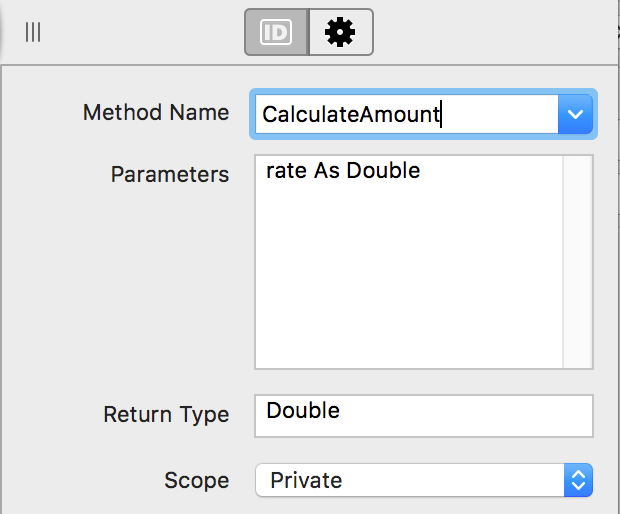
Methods can be added to project items, including classes (including Desktop Windows, Web Pages, Mobile Screens) and modules. To add a method, choose Insert -> Method from the menu or toolbar when the appropriate project item is selected. This adds the method, displays a blank code editor and displays the Inspector where you can set the properties for the method:
Method Name
The name of the method. Just like variables, methods are given names to describe them and the same naming rules apply.
Parameters
Parameters are values that you pass to the method that it can then use within the method as if they were variables. Parameters are separated by commas and are declared similarly to how you use the Var statement:
value As Integer
value As Integer, name As String
Return Type
Methods that do not specify a return type are called Subroutines (or Procedures in some other languages). Methods that specify a return type are called Functions. The Return Type can be any valid data type, including classes.
Scope
Scope indicates what parts of your code can call the method. Choices are Public, Protected and Private.
Global methods (used with Modules) can be called from anywhere in your code with no restrictions.
Public methods (used with Classes) can be called from anywhere in your code with no restrictions.
Protected methods when used in classes can be used only within the class itself or within a subclass. When used with modules, the method can be used only in conjunction with the module name.
Private methods can only be called by the module or class that contains the method.
Note
Scope is indicated in the Navigator via the background color of the item. An item that is public will have no background color. An item that is Protected has a yellow background. An item that is Private will has a red background.
The method signature (its name, parameters and return type) also appears at the top of the Code Editor for reference.
Using methods
To use a method you specify its name, optionally prefixed by the module or class instance name (depending on how the method is defined).
An example of a method you have used before is the ToString method on the number data types:
Var i As Integer = 42
Var s As String = i.ToString
Passing parameters to methods
Some methods are called simply with their name. But some methods require additional information or values. This information that is passed to a method is called a parameter. A method can have any number of parameters.
If you have a Dictionary containing a value for key "Name", then this method call removes the key and value from the Dictionary:
myDictionary.Remove("Name")
The value "Name" was passed as a parameter to the Remove method.
Methods define their parameters and specify the types for them. A method can have any number of parameters. When passing multiple parameters, separate each one with a comma.
The Lookup method on a Dictionary takes two parameters and can be used to get a value for a key and if not available, provide a default:
Var value As String = myDictionary.Lookup("Name", "Unknown")
In these examples, the parameters have been passed as text literals, but you can also pass variables and constants as parameters instead:
Var key As String = "Name"
Var default As String = "Unknown"
Var value As String = myDictionary.Lookup(key, default)
As with variable assignment, the parameters you pass to the method must match the types of the parameters as declared on the method.
Passing arrays as parameters
An array can be passed as a parameter in a call to a method or function. You can pass both one and multi-dimensional arrays. To specify that a parameter is a one-dimensional array, put empty parentheses after its name in the declaration. For example:
names() As String
can be used in the declaration when you want to pass an array of strings to the method. Since you do not need to specify the number of elements in the array to be passed, you can pass a different number of elements at different places in your code. When you pass an array to the method or function, omit the parentheses in the array. For example:
PrintLabels(allNames)
PrintLabels is the name of the method that accepts the text array as its parameter.
You can pass multi-dimensional arrays without specifying the number of elements in each dimension, but you need to indicate the number of dimensions. Do this by placing one fewer commas in the parentheses than dimensions. For example, if names were a two-dimensional String array, you would declare the array as a parameter in the following manner:
names(,) As String
When you pass a multi-dimensional array to a method or function, you can include the parentheses but not any commas:
PrintLabels(allNames())
Returning values from methods
Some methods return values. These methods are also called Functions. When a method returns a value, the value is passed back from the method to the line of code that called the method. For example, the method System.Ticks returns the number of ticks (a tick is 1/60th of a second) that have passed since you turned on your computer. You can assign the value returned by a method the same way you assign a value to a variable. In the example below, the value returned by Ticks is assigned to the variable elapsed:
Var elapsed As Double
elapsed = System.Ticks
Some methods require parameters and return a value. You saw this earlier with the Lookup method for a Dictionary:
Var value As String = myDictionary.Lookup("Name", "Unknown")
The above method takes two parameters and returns a value.
You can also directly pass methods that return values to other methods. This code finds "are" in the source String:
Var source As String = "Hello, how are you?"
Var search As String = "You are"
Var position As Integer
position = source.IndexOf(search.Right(3))
For a method to return a value, you just have to specify a Return Type in the method properties of the Inspector. For example, set the Return Type to "Integer" to return an Integer.
A method can return any type, from the intrinsic data types to any class or other type you have created yourself.
Methods can also return arrays of any type. To indicate that the return type is an array, add the double parenthesis to it. To return an Integer array set the Return Type to "Integer()".
Passing parameters by value and by reference
By default, you pass values to a method by value. When you do so, the method receives a copy of the data that you pass allowing the method to modify it without affecting the original value.
Passing by value
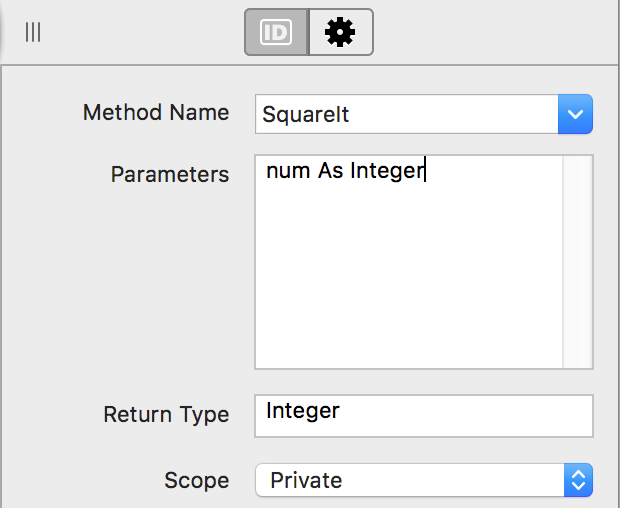
Parameters passed by value are essentially treated as local variables inside the method — just like variables that are created using the Var statement. This means that you can modify the values of the parameters themselves rather than first assigning the parameter to a local variable. For example, if you pass a value to the Integer parameter “x”, you can increment or decrement the value of x rather then assigning the value of x to a local variable that is created using Var.
This example modifies the parameter and returns the result:
Function SquareIt(num As Integer) As Integer
num = num * num
Return num
End Function
The original value passed to the method is not changed, as shown in this code:
Var value As Integer = 10
Var result As Integer
result = SquareIt(value)
' value remains 10
' result is 100
Passing by reference
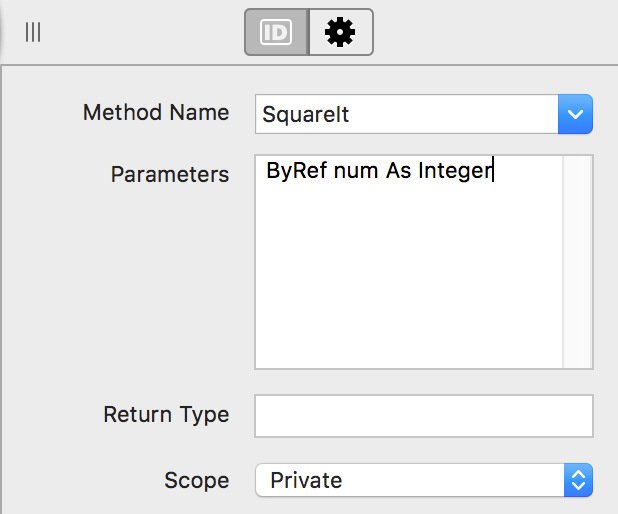
When you write your own methods, you have the option of passing information by reference by using the ByRef statement. The practical advantage of this technique is that the method can change the values of each parameter and replace the values of the parameters with the changed values. When you pass parameters by value, you can't do this because the parameter only represents a copy of the data itself.
When you pass information by reference, you actually pass the object itself rather than a copy of it.
Note
There are basically two kinds of variables: value types, which hold the value directly (e.g., an Integer or Double), and reference types, which contain too much data to store in a single memory location. Instead, a reference is a pointer to a larger block of data — such as an array or a class instance.
Arrays and objects are reference types. So when you don't use ByRef, what gets passed for a value type is a copy of the value. For a reference type, a copy of the reference is passed. Since it's still a reference, you can access and modify the original array or object through it.
Now, let's add ByRef into the picture:
With a value type, ByRef passes a reference to the original value — so you can modify the original directly.
With a reference type, it's essentially the same — you get a reference to the reference, allowing you to change what the original reference points to.
Here the SquareIt example from above written to use ByRef:
Sub SquareIt(ByRef num As Integer)
num = num * num
End Sub
Since this modifies the parameter value and does not return a value, it is called like this:
Var value As Integer = 10
SquareIt(value)
' value is now 100
Optional parameters and default values
There are two ways to make a parameter optional, both of which you do at the time you write the method declaration:
Assign a value to it in the declaration
Use the Optional keyword in the declaration
The value you assign can be a literal value or a standard constant (not a localized string).
The best way to make the parameter's explicit status clear is to use the Optional keyword. This modifier precedes the parameter name and may be used with or without a default value. When the caller omits passing this parameter, the parameter receives the assigned default value or the default value for its data type if no default value was assigned in the method declaration. For example:
MyMethod(a As Integer, b As Integer, Optional c As Integer)
The parameter c is optional. If it is omitted in the method call, then it gets the default value of Integer, which is 0.
If you want a different default value, you assign it to the parameter:
MyMethod(a As Integer, b As Integer, Optional c As Integer = 5)
Now if the parameter c is omitted in the method call, then it gets the value 5.
Lastly, you can omit the Optional keyword from the declaration if you specify a default value:
MyMethod(a As Integer, b As Integer, c As Integer = 5)
With any of the the above method declarations, you can call the method with only 2 of the 3 parameters:
MyMethod(3, 4)
Identifying the currently executing method name
For logging purposes, it can often be useful to know the name of the currently running method. Use the CurrentMethodName method in your code to get the name of the currently running method:
MessageBox(CurrentMethodName)
Setters
A value passed to a method is normally supplied in parenthesis following the method name, such as:
MyMethod(10)
Sometimes it is preferred to have the method call behave differently so that you assign the parameter like this:
MyMethod = 10
This is called a Setter because it looks like you are setting the MyMethod value to 10 rather than passing 10 as a parameter to MyMethod. You can enable this alternative syntax by using the Assigns keyword in the parameter declaration:
Sub MyMethod(Assigns value As Integer)
Now you call MyMethod like this:
MyMethod = 10
You can have more than one parameter with this technique but only the last parameter can have the Assigns keyword. The other parameters are passed as before. For example:
Sub MyMethod(desc As String, Assigns value As Integer)
To call this method:
MyMethod("test") = 10
Events
Events are a special type of method commonly used with control classes. But they can also be implemented in your own non-control classes as well. Events can be called only by the class that declares the event. Generally speaking, events are used to provide a way for subclasses to provide additional functionality.
You don't need to worry about Events right now. Both subclasses and events are described in more detail in other topic pages.