Introduction to app localization
Localization is the process of making your app display appropriately for a specific country or region. For example, this may involve:
Displaying text in a different language
Formatting numbers using alternative thousands and decimal separators
Formatting dates using an alternative date pattern
Formatting currency using a specific currency symbol
Text localization with localizable strings
You localize text for your apps using localizable strings created with the Constant Editor. These are a special form of a constant that is added to a project item such as a module, window, web page or class. Only constants of type String or Text can be marked as localizable. When the constant is localizable, it can have different values to use for localization.
To identify a constant as localizable, create a String or Text constant and then enable the Localizable switch in the Inspector. A recommended approach is to create a separate module for these localizable strings, but you can put them anywhere that is publicly accessible.
Localized strings are not actually constants, so they cannot be used in places where a standard constant can be used, such as method parameter default values or with conditional compilation.
You can enter different localized values for the string based on platform and language. Do this using the Constant Editor.
Use the “+” and “-” buttons to add or remove a specific localization. You can choose the Platform and the Language for which to specify a value.
When the user runs an app, the language specified on their system is used to look up the localized value of the string to use.
Localized Strings created in this manner can then be used as the Text or Caption of controls so that the control displays the appropriate localized value. To use a localized string as the Text or Caption, you prefix it with the “#” character when entering it in the appropriate property. If you have a module called LocalizedStrings and have a protected localized string called kWelcomeMessage, then you add it to a Label as the Text property like this:
#LocalizedStrings.kWelcomeMessage
You can also refer to the localized string in your code just as you would a constant, but since this is a special type of constant, you can also specify which localization you want to use. You do this by supplying the language code (usually two-character) and optionally the region code as a parameter to the localized string name. For example, if you had a localized string called kHello that had localizations for both French (“Bonjour”) and English (“Hello”), you could force the French version to be displayed by doing this:
Var s As String
s = kHello("fr") // s = "Bonjour"
s = kHello("en") // s = "Hello"
s = kHello("en_UK") // s = "Welcome"
And you could grab the default value like this, although you're better off getting the specific language value you want:
s = kHello("default")
Building the localized app
On the Shared Build Settings, there is a Language property in the Build section of the Inspector. This property determines the language that is used by any localized strings that have “Default” as the language.
It is important that you select a specific language in this build setting. If you also leave this setting at “Default”, you will run into confusion if the project file is shared with people that do not have the same system language as you.
For example, if you leave both the localized string language and build language as “Default” then “Default” becomes “English” for users that build with an English system and becomes “French” for users that build with a French system.
To prevent this confusion, always at least choose a specific language in the Build setting. Alternatively, don 't use “Default” for your localized strings and instead always choose the exact language for them.
Lingua
If you have a lot of text to localize, it can get tedious to enter all the values using the Constant Editor. The Lingua app is used to simplify the localization process. With Lingua you can localize your app strings outside of the project by using the localized strings you have already created.
Refer to the Lingua topic to learn more.
Date and number localization
You can localize how dates and numbers are displayed by using the Locale class. The shared Current method returns the locale that is specified in the system or device settings. You can then use this with the ToString method on DateTime, Double and Integer to display values formatted properly for the locale.
This code (in a Label's Opening event handler), displays the current date:
Me.Text = DateTime.Now.ToString(Locale.Current, DateTime.FormatStyles.Long, DateTime.FormatStyles.None)
On an US English system, this displays: January 9, 2017
On a French system, this displays: 9 janvier 2017
This code (also in a Label's Opening event handler), displays a formatted number:
Var num As Double = 1234.56
Me.Text = num.ToString(Locale.Current, "#,###.##")
On an US English system, the number displays like this: 1,234.56
On a French system, it displays like this: 1 234,56
Localizing web apps
Web apps can also localize their text using dynamic constants, but the language that is displayed is determined by the language setting in the browser being used to access the web app. More specifically, it is using the constant value that is most appropriate for the HTTP header language setting of the current session.
There are also several properties of the WebSession that are helpful for localization:
LanguageCode
LanguageRightToLeft
In addition, if you select Session in the Navigator and then open the Inspector, you can directly localize the messages that appear in dialogs when the connection is interrupted disconnected or when the user must confirm they want to leave the session. You can also use constants instead to provide localization in multiple languages.
Finally, WebApplication has an HTMLHeader property for which you can provide a value using your own dynamic constants.
Dates and numbers
In desktop apps, you can use Locale to get the user's locale for formatting dates and numbers. However, in a web app this value returns the locale used by the web server rather than the locale of the current user session.
To display dates formatted in the locale of the user session, you need to get the LanguageCode from WebSession and use that to create a locale that you can then use to display the date. This code (in a WebLabel Shown event handler) gets the language code for the user session and then uses it to display the current date:
Var langCode As String = Session.LanguageCode.DefineEncoding(Encodings.UTF8).ToString
Var locale As New Locale(langCode)
Me.Text = DateTime.Now.ToString(locale, DateTime.FormatStyles.Long, DateTime.FormatStyles.None)
When the browser is set to English, this displays: January 9, 2017
When it is set to French, this displays: 9 janvier 2017
Use the same technique with number formatting. This code (in a WebLabel Shown event handler) gets the language code for the user session and then uses it to display a formatted number:
Var langCode As String = Session.LanguageCode.DefineEncoding(Encodings.UTF8).ToString
Var locale As New Locale(langCode)
Var num As Double = 1234.56
Me.Text = num.ToString(locale, "#,###.##")
When the browser is set to English, the number displays like this: 1,234.56
When it is set to French, it displays like this: 1 234,56
To get locale information that is more specific than just the language, you should check the value of the "Accept-Language" HTTP header:
Var langHeader As String = Session.Header("Accept-Language")
Which returns both the language and region code in a format like this: en-US.
Note that there may be more than one value returned in this header so you may need to parse it to ensure you get the value you actually want.
Changing the language
Windows
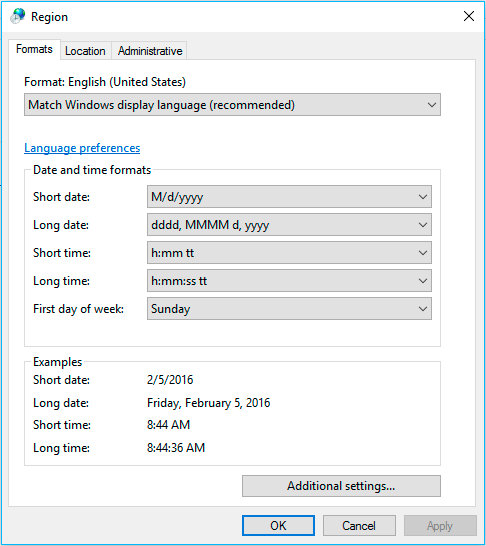
To use a different language on Windows, you need to change the Language setting on the Format tab of the Region control panel. Changing the Language on the Keyboard and Languages tab will not affect your applications.
For Windows 10:
Open Settings
Click Time & Language
In "Related Settings", click "Additional date, time & regional settings"
In the Region section, click "Change location"
Click the Formats tab to display the drop-down where you can change the language
macOS
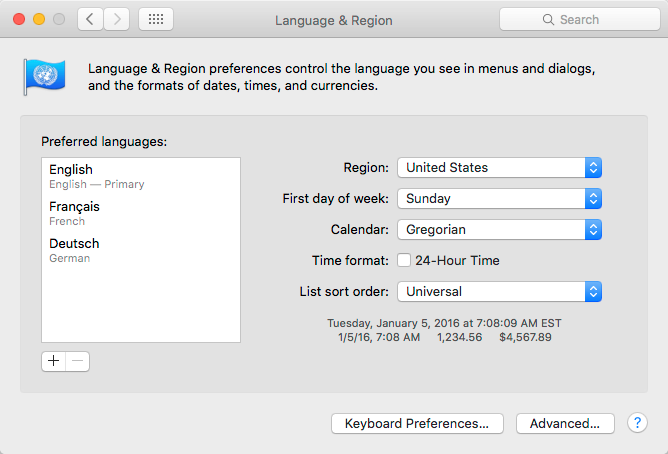
To change the language on Mac, open System Preferences and select "Languages & Region" (top row). In the list of Preferred languages on the left, you can drag the language you want to be the primary language to the top.
Linux
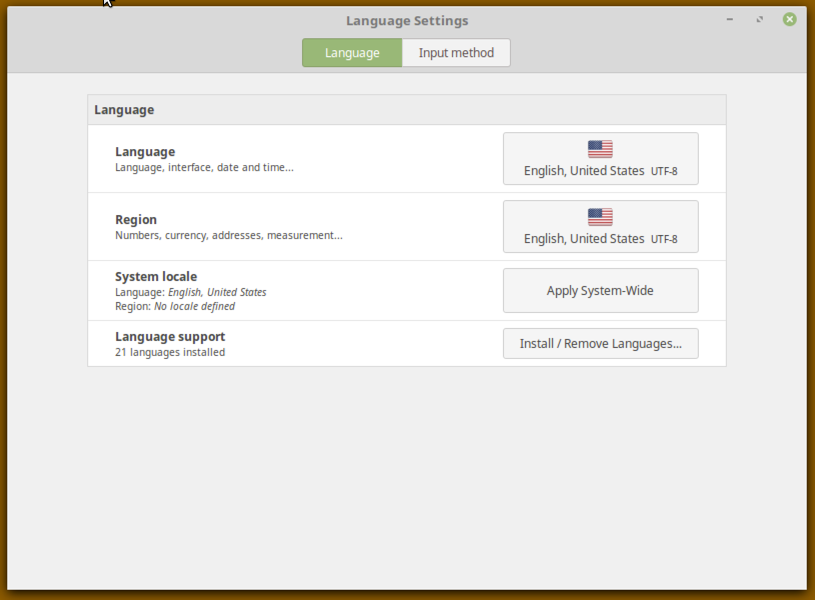
The specific way to change the language and region varies by Linux distribution. On Linux Mint 18, you can make the changes by going to Preferences > Languages and making the appropriate changes.
iOS
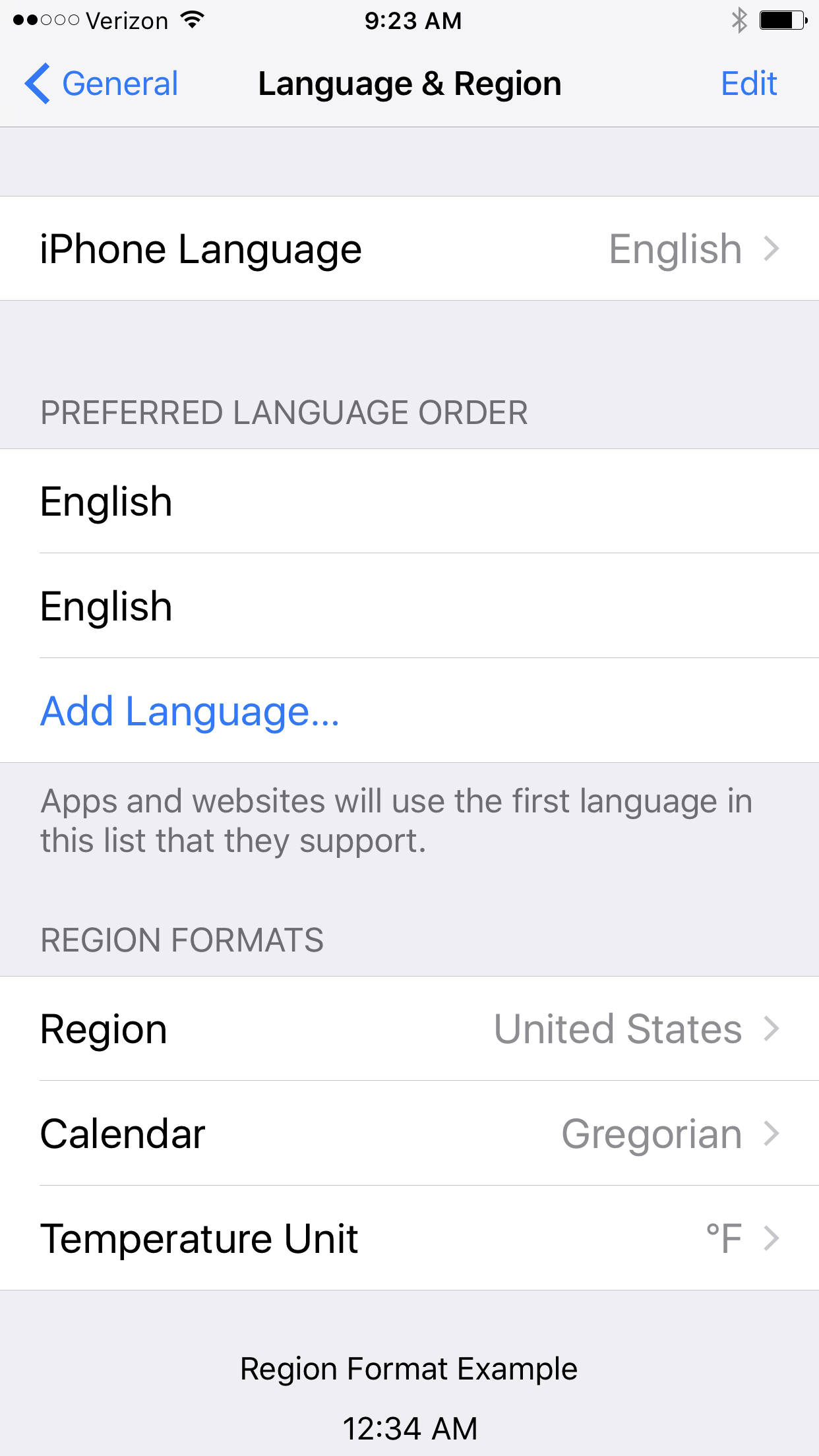
To change the language on iOS, open the Settings app and select General, followed by "Language & Region". On this screen you can change the preferred language order and region formats.
Web browsers
To see a web app in a different language, you'll need to change the settings for the browser that is connecting to the web app. This varies by browser.
Browser |
Language Setting |
---|---|
Edge |
Uses the system settings in Settings / Control Panel. |
Internet Explorer |
Go to Internet Options. In the General tab click the Languages button to display the screen to set the language. |
Safari |
Uses the system settings in System Preferences. |
Chrome |
Go to Settings and then click Advanced settings. Look for the Languages section. |
Firefox |
Go to Preferences, select Content and look for the Languages section. |
See also
Using the Lingua App to localize your app, Constant Editor topics