Conditional compilation
In the process of creating an application that runs on multiple platforms, you may find that you have some code that is only needed on a single platform. An example of this might be code that saves preferences. On Windows you might want to use the Registry, but on Mac you might want to use a plist. You can handle these special cases using conditional compilation.
With conditional compilation, you are telling the compiler to include or exclude specific parts of your code depending on what is platform and/or target being compiled. This is done using the #If command in conjunction with #Else, #ElseIf and #Endif.
Usage
You can use these commands along with any constant to selectively include or exclude code when building. There are many built-in compiler constants you can use with conditional compilation. Some commonly used constants include:
DebugBuild
TargetWindows
TargetMacOS
Target64bit
For example if you have code that should only be included when you are running in debug mode from the IDE, you would do this:
#If DebugBuild Then
// Activate logging
App.Logging = True
#Else
App.Logging = False
#Endif
This code could be used to handle saving preferences differently based on platform:
#If TargetWindows Then
// Use Registry
SavePreferencesToRegistry
#ElseIf TargetMacOS Then
// Use a plist
SavePreferencesToPList
#ElseIf TargetLinux Then
// Use generic XML
SavePreferencesToXML
#Endif
You can even use your own constants, such as this to check if a beta has expired:
#If App.kBetaBuild Then
// Check if the beta has expired
If DateTime.Now.Year > 2022 Then
Quit
End If
#Endif
For more information, refer to .
Selecting a target for a class/method in Inspector
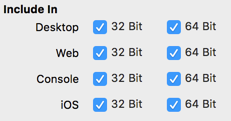
You can indicate that a particular project item (excluding Windows, Web Pages and iOS Views) should only be included for certain project types. For example, you may have a class that you only want to include when you are creating a web app. You do this using the “Include In” properties in the Advanced tab of the Inspector (the are often referred to as Compatibility Flags). To get to the Advanced tab, click the gear button at the top of the Inspector. This shows the advanced options. The "Include In" section has properties for the target (Desktop, Web, Console, IOS) and architecture (32-bit or 64-bit). By default everything is checked, but you can check any combination.
When your project is being built, the project item gets excluded from any target/architecture that does not match what is checked.
You can also set “Include In” for methods, properties, constants, event definitions, enums, structures, delegates and anything else you can add to a project item.
See also
#If...#Endif commands; Constants topic