Secure web login screens
When creating login screens for your web apps, it is important that that the user's login information is protected. By default, a hosted web app cannot transmit information securely. This means that the username or password could be read by anyone watching network traffic. The solution to this is to use SSL to encrypt the traffic between the web browser and the web app. In order to use SSL, you'll need to install a certificate on your web server and set up your app to use it.
Refer to SSL for Web Apps for information on how to set up a web app to use SSL. Use Introduction to Xojo Cloud for simple 1-click SSL setup.
Designing the login screen
A typical login screen prompts for a username of some kind (maybe an email address or some other unique identifier) and a password. A good strategy for creating your login screen is to use a modal dialog on an otherwise empty page. This allows you to deal with the complexities of the login process without any other code possibly getting in the way.
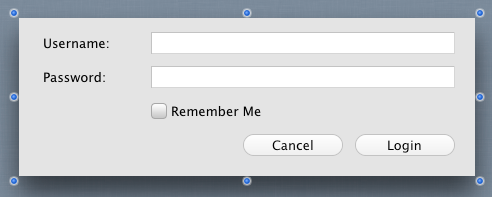
Create the layout as a modal dialog (LoginDialog) and then add it to a web page (called LoginPage). Drag LoginDialog to the page (name it MyLoginDialog) and in the Shown event for the page you can display the dialog like this:
MyLoginDialog.Show
Displaying the login page
Because SSL connections are noticeably slower than non-SSL connections (because no caching is allowed, among other things), you likely won't want your entire web application to be using SSL. What you really want is for your app to switch to SSL when it gets to the login screen.
If you've created your login page as a web page called LoginPage that displays a web dialog in its Shown event, you can use code like this to check if the connection is secure in the LoginPage.Opening event:
// Make sure the user is connecting securely,
// If not have the browser load the application securely
If Not Session.Secure Then
Var host As String = Session.Header("Host")
Var url As String = "https://" + host
System.GotoURL(url)
End If
This reloads your web app and securely takes the user to the login page.
To display the login page by default, you have the Session do it. In the WebSession.Opening event add this code which displays the login page whenever a secure connection is made to the web app:
If Self.Secure Then
LoginPage.Show
End If
Remember the user
If you look at the screen shot above, there is a "Remember Me" checkbox. Typically these are used to remember the UserID to help jog the end-user's memory about what their password might be. This function uses a browser feature called Cookies which stores a snippet of information on the computer where the user is (assuming cookies are enabled, of course).
Note: It is best to not remember the user's password because if this box gets checked on a public computer (like a library or internet cafe) the user's account could be compromised.
To make the checkbox work, you need two pieces of code in LoginDialog.Shown:
If Session.Cookies.Value("username") <> "" Then
UserNameField.Text = Session.Cookies.Value("username")
RememberMeCheck.Value = True
PasswordField.SetFocus
End If
This code first checks to see if the "username" cookie has been set, and if it has, places the value into the Username TextField, checks the RememberMe checkbox and sets the focus to the Password field (mostly a convenience so the user doesn't need to do that manually).
Then create a DoLogin method on LoginDialog that checks the value of the RememberMe checkbox and either saves the username to the cookie or removes the cookie:
If RememberMeCheck.Value Then
Session.Cookies.Set("username", UserNameField.Text)
Else
Session.Cookies.Remove("username")
End If
// Now validate the credentials and if they are OK, you can close the dialog
// and show the main page.
If ValidateCredentials Then
Self.Close
MainPage.Show
Else
MessageBox("The username/password combination does not match.")
End If
Lastly, in the Pressed event of the Login button call the DoLogin method:
DoLogin
Example project
Example Projects/Web/SecureLoginExample
See also
Dialog Boxes, SSL for Web Apps topics