Working with color
Colors are specified using the Color data type. There are several ways to define a color, using several different formats including RGB, HSV and CMY.
RGB
RGB (red, green, blue) is the most common way to specify a color. With RGB you specify the red, green and blue components of a color using an Integer ranging from 0 to 255. This can be done using the RGB method or a hexadecimal color literal.
For example, to create a red color using the RGB method:
Var red As Color = Color.RGB(255, 0, 0)
You can also specific a Color using a color literal, which consists of the characters "&c" followed by the hexadecimal value RGB color in this format: &cRRGGBB
. This specifies red using a color literal:
Var red As Color = &cff0000
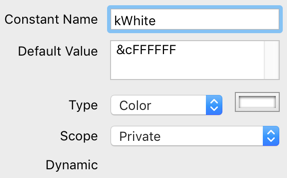
You can also use these color literals to create constants on a project item.
HSV
The HSV method specifies a color using the hue, saturation and value which are each represented by Doubles between 0 and 1.
Var m c As Color = Color.HSV(0.8, 0.5, 0.75)
CMY
The CMY method specifies a color using the cyan, magenta and yellow components of the color, also as Double values between 0 and 1. CMY is a global function s you code it like this:
Var c As Color = Color.CMY(0.35, 0.9, 0.6)
Color constants
The Color data type has several constants for commonly used colors. These constants are: Black, Blue, Brown, Clear, Cyan, DarkGray, Gray, Green, LightGray, Magenta, Orange, Purple, Red, Teal, White and Yellow. This specifies red using the constant:
Var red As Color = Color.Red
Inspector
When using the Inspector with Color properties you will see a small "color box" next to the color property. You can click it to display the OS color chooser to choose a color for the property.
Transparency
Transparency indicates how much of the background shows through the color. When you use any of the techniques described above, the color value is not transparent at all and is referred to as opaque. If you want to have a color with transparency, use the RGB or HSV methods which have an additional parameter where you can supply an alpha value from 0, the default of opaque, to 255 which is fully transparent. The alpha parameter is optional with the CMY method. Here are examples:
Var c1 As Color = Color.RGB(255, 0, 0, 255)
Var c2 As Color = Color.HSV(0.8, 0.5, 0.75, 50)
Var c3 As Color = Color.CMY(0.8, 0.5, 0.75, 50)
For the &c color literal, you can specify the transparency as an additional hexadecimal value. This would be a fully transparent red color:
Var c As Color = &cFF0000FF
Usage
All of the above techniques return a Color that you can then use with various color properties available on classes.
For example, in Paint event of a Canvas the DrawingColor property of the Graphics object is set to blue-is so the text drawn will be in that color:
g.DrawingColor = Color.RGB(9, 13, 80)
g.DrawText("Hello World", 50, 50)
Transparency example code
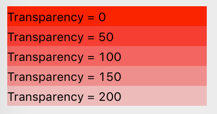
This code draws sample color patches in a Canvas at varying levels of transparency. The code is in the Paint event:
Var red As Color
For i As Integer = 0 To 4
red = Color.RGB(255, 0, 0, i * 50)
g.DrawingColor = red
g.FillRectangle(0, i * 20, 200, 20)
g.DrawingColor = &c000000
g.DrawText("Transparency = " + Str(i * 50), 0, i * 20 + 15)
Next
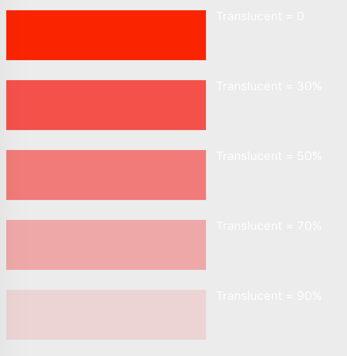
This code code (in the Paint event of a Canvas) uses the CMY function to draw sample color patches in a Canvas with varying levels of transparency:
g.DrawingColor = Color.CMY(0.0, 1.0, 1.0) // red, no transparency
g.DrawRectangle(0, 0, 200, 50)
g.FillRectangle(0, 0, 200, 50)
g.DrawingColor = Color.CMY(0.0, 0.0, 0.0)
g.DrawText("Translucent = 0", 210, 10)
g.DrawingColor = Color.CMY(0.0, 1.0, 1.0, 76) // transparency = 0.3
g.FillRectangle(0, 70, 200, 50)
g.DrawingColor = Color.CMY(0.0, 0.0, 0.0)
g.DrawText("Translucent = 30%", 210, 80)
g.DrawingColor = Color.CMY(0.0, 1.0, 1.0, 127) // transparency = 0.5
g.FillRectangle(0, 140, 200, 50)
g.DrawingColor = Color.CMY(0.0, 0.0, 0.0, 0)
g.DrawText("Translucent = 50%", 210, 150)
g.DrawingColor = Color.CMY(0.0, 1.0, 1.0, 178) // transparency = 0.7
g.FillRectangle(0, 210, 200, 50)
g.DrawingColor = Color.CMY(0.0, 0.0, 0.0, 0)
g.DrawText("Translucent = 70%", 210, 220)
g.DrawingColor = Color.CMY(0.0, 1.0, 1.0, 229) // transparency = 0.9
g.FillRectangle(0, 280, 200, 50)
g.DrawingColor = Color.CMY(0.0, 0.0, 0.0, 0)
g.DrawText("Translucent = 90%", 210, 290)
Selecting a color with the Color Picker
If you want to let the user choose a color at runtime you can use the Color Picker by calling the Color.SelectedFromDialog method. The following code displays the Color Picker:
Var c As Color
Var b As Boolean
b = Color.SelectedFromDialog(c, "Choose a color")
If b Then // user chose a color
// do something with the color (c)
End If
If the user cancels out of the Color Picker dialog box, the boolean variable, b, is False; otherwise, the selected color is returned in the color object parameter, c which you can then use to assign to a color property.
In the macOS color pickers, you click on a color and a sample of the color appears in the patch area at the top of the screen. When you click OK, the RGB values appear.
The Windows version of the Color Picker uses only one format. You can either select one of the predefined colors or click the Define Custom Colors button to display the “advanced” color picker, which depicts colors on a continuum and lets you specify the color using either the RGB or HSV models. Select a color by clicking on a point in the color spectrum or enter values in the RGB or HSV areas. Click Add to Custom Colors to add the custom color to one of the Custom Color samples on the left side of this dialog.
In the Code Editor you can also right-click and select "Insert Color" to display the Color Picker so that you can choose a color. When you choose a color, the literal color hex value is placed at the current position of the cursor in the Code Editor.
See also
Color data type; Color Groups, Color.SelectedFromDialog function