Drawing with Vector Graphics
A vector graphic (as opposed to a bitmap graphic) is composed entirely of primitive objects — lines, rectangles, text, circles and ovals, and so forth — that retain their identity in the graphic. They can be resized to display at any size and do not pixelate or otherwise “decompose” like bitmap graphics can. The Object2D class is the base class for all the classes that create primitive objects, which include: ArcShape, CurveShape, FigureShape, OvalShape, PixmapShape, RectShape, RoundRectShape and TextShape. Each of these classes allow you to specify borders, fill and fill colors, rotation, scale and positioning.
Drawing and displaying a vector object
You draw a single vector object simply by instantiating it and specifying its properties. For example, the following code defines a RoundRectShape:
Var r As New RoundRectShape
r.Width = 120
r.Height = 120
r.Border = 100
r.BorderColor = Color.RGB(0, 0, 0) // black
r.FillColor = Color.RGB(255, 102, 102)
r.CornerHeight = 15
r.CornerWidth = 15
r.BorderWidth = 2.5
The only problem with this is that the shape doesn 't appear anywhere. It's just “defined” — ready for your use. You need to add a command to draw the vector object in another object. As you learned in the previous section, a DesktopCanvas is a great place to draw stuff.
Use the DrawObject method of Graphics to draw Vector graphics. Adding DrawObject to the above code and adding it to the Paint event of a Canvas control:
Var r As New RoundRectShape
r.Width = 120
r.Height = 120
r.Border = 100
r.BorderColor = Color.RGB(0, 0, 0) // black
r.FillColor = Color.RGB(255, 102, 102)
r.CornerHeight = 15
r.CornerWidth = 15
r.BorderWidth = 2.5
g.DrawObject(r, 100, 100)
Combining vector objects
You can also create composite vector graphics objects that are made up of several individual vector graphics objects. The composite object is a Group2D object—it's just a group of Object2D objects. Use the Append or Insert methods of the Group2D class to add individual vector graphic objects to the Group2D object. When you are finished, draw the object using one call to the DrawObject method.
To illustrate this, take the above code and add a second RoundRectShape object offset from the original by 20 pixels. Use AddObject
to add the two RoundRectShapes to the Group2D object and then draw it in the Canvas:
Var r As New RoundRectShape
r.Width = 120
r.Height = 120
r.Border = 100
r.BorderColor = Color.RGB(0, 0, 0) // black
r.FillColor = Color.RGB(255, 102, 102)
r.CornerHeight = 15
r.CornerWidth = 15
r.BorderWidth = 2.5
Var s As New RoundRectShape
s.Width = 120
s.Height = 120
s.Border = 100
s.BorderColor = Color.RGB(0, 0, 0) // black
s.FillColor = Color.RGB(255, 102, 102)
s.CornerHeight = 15
s.CornerWidth = 15
s.BorderWidth = 2.5
s.X = r.X + 20
s.Y = r.Y + 20
Var group As New Group2D
group.AddObject(r)
group.AddObject(s)
g.DrawObject(group, 100, 100)
Combining bitmap and vector graphics
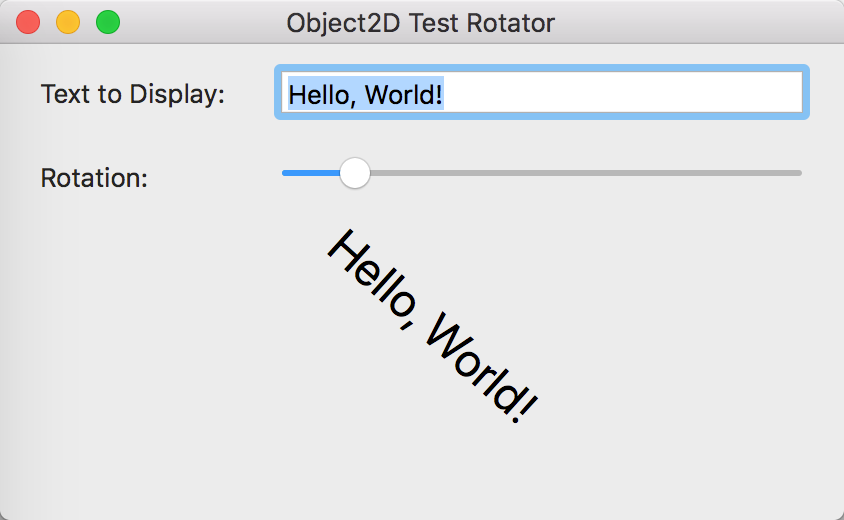
You can combine both bitmap and vector graphics by first loading the bitmap graphic into a vector object using the PixmapShape class. You then group this with your other vector objects to create a single image. This example combines a bitmap graphic that was added to the project with a TextShape:
Var px As PixmapShape
px = New PixmapShape(PictureName)
Var s As TextShape
s = New TextShape
s.Y = 70
s.Value = "Hello, world!"
s.FontName = "Helvetica"
s.Bold = True
Var d As New Group2D
d.AddObject(px)
d.AddObject(s)
g.DrawObject(d, 100, 10)
Saving and loading vector graphics
Vector graphics can be saved using the Save method of the Picture class. Vector graphics are stored as PICT files on macOS and as EMF files on Windows.
Note
Saving and Loading vector graphics is not supported on Linux.
Since you need a Picture object to call save, you should draw your vector graphics to a picture first rather than directly to the Canvas. This gives you the Picture that you can save and allows you to also draw the picture to the Canvas if needed. In this example, SavePicture is a Picture property on the window:
Var r As New RoundRectShape
r.Width = 120
r.Height = 120
r.Border = 100
r.BorderColor = Color.RGB(0, 0, 0) // black
r.FillColor = Color.RGB(255, 102, 102)
r.CornerHeight = 15
r.CornerWidth = 15
r.BorderWidth = 2.5
SavePicture.Graphics.DrawObject(r, 100, 100)
g.DrawPicture(SavePicture, 0, 0) // Draw SavePicture in the Canvas
To save this picture, add a button called Save to the window and use this code:
Var file As FolderItem
If TargetMacOS Then
file = FolderItem.ShowSaveFileDialog("", "vector.pict")
Else
file = FolderItem.ShowSaveFileDialog("", "vector.emf")
End If
If file <> Nil Then
SavePicture.Save(file, Picture.SaveAsDefaultVector)
End If
To load a vector image, you use the OpenAsVectorPicture method of FolderItem. This code prompts the user to select a vector image and then displays it in an ImageWell:
Var file As FolderItem
file = FolderItem.ShowOpenFileDialog("")
If file <> Nil Then
Var p As Picture
p = Picture.OpenVector(file)
If p <> Nil Then
VectorWell.Image = p
End If
End If
See also
Object2D class