Enumerations
An enum or enumeration is a data type consisting of a set of named values, which are called elements.
Creating your own enumerations
Enumerations can be added to project items such as classes (including Windows, Web Pages, iOS Views) and modules. To add an enumeration, choose Insert > Enumeration from the menu or toolbar when the appropriate project item is selected. This adds the Enumeration, displays a blank Enumeration Editor and displays the Inspector where you can set the properties for the enumeration.
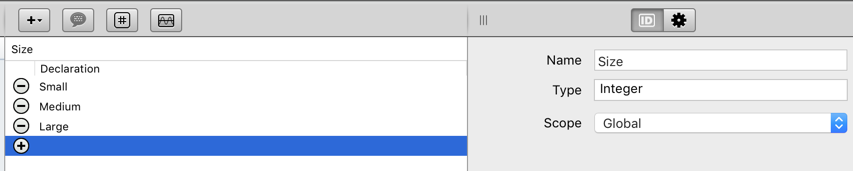
Name
The name of the enumeration.
Type
Enumerations are always an Integer type and default to “Integer”. You can change the type to other Integer types (such as UInt64) should you need to use larger values for the enumeration elements.
Scope
Scope indicates what parts of your code can call the method. Choices are Public, Protected and Private.
Public enums can be called from anywhere in your code with no restrictions.
Protected enums have some restrictions, which vary depending on where the enum is located (class or module).
Private enums can only be called by the module or class that contains the enum.
In the Enumeration Editor, you use the “+” icon to add a new enumeration element to the set. Use the “-” button to remove an element from the set. When you add an element, you also give it a name. The name is used to refer to the value. You can edit the name by clicking on it once to select it and a second time to edit it.
Note: The enumeration name cannot be left blank.
Although enumeration elements are integers behind the scenes, you don 't normally worry about the integer values. However, should you need to assign a specific integer value to an enumeration item, you can do so by assigning it as part of the name like this:
EnumElement = 10
To use an enumeration in code, you refer to the container of the enumeration, the enumeration name and then the enumeration element name. When an enumeration is in a class, refer to it using the class name and not the name of the instance variable or property.
This code assigns an element to an enumeration:
Var pictureSize As Module1.Size // Size is an enumeration
pictureSize = Module1.Size.Large // Large is an element on Size
If you find you need the actual Integer value stored by the enumeration, then you have to convert (cast) it to an Integer first:
Var pictureSize As Integer
pictureSize = Integer(Module1.Size.Large)
It is not possible to convert an integer value back to an enumeration automatically so you'll need to use a condition (such as a Select Case) and do it like this:
Var picSize As Module1.Size
Select Case pictureSize
Case 0
picSize = Module1.Size.Small
Case 1
picSize = Module1.Size.Medium
Case 2
picSize = Module2.Size.Large
End Select
Binary enumerations
You can create enumerations that support binary operations by enabling Binary in the Inspector. See the Enumerations data type for a list of supported operations. The binary values will be assigned automatically when you create the enumeration members. Binary enumerations are only available in modules. They must be of type Integer.
See also
Enumeration data type