Working with fonts
A font defines how text is displayed on the screen. Typically a font has a family, such as "Helvetica, Times, Courier, etc." and a corresponding size that is usually measured in points.
For most of your apps you will want to use the system standard font size and point size so that things are as readable as possible and so that any changes the user makes to accessibility settings (to alter font readability) are properly used by your app.
Still, there may be cases when you need to choose a specific font for your text display. This topic describes how that works for desktop, web and iOS projects.
Desktop fonts
You have the ability to set the font, font size, and font style of many of the objects and controls in your app. Text Areas support multiple fonts, styles, and sizes (collectively referred to as styled text) and List Boxes support multiple styles as well. Desktop controls that use a single font have a TextFont property that you can set by assigning it the name of the font you want used to display text for the control. Additionally, many controls also have checkboxes to specify if the font should be Bold, Italic or Underlined.
Text Areas have a TextFont property but they can also display multiple fonts and all kinds of formatted text. For information on this, refer to the Creating Styled Text topic.
System and SmallSystem fonts
The System font is the font used by the system software as its default font. It's the font used for the menus as well. This font varies by operating system and the user's preferences.
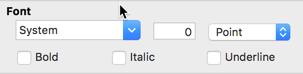
If you want text to be shown using the user's System font, use the name "System" as the font when you assign it. You can enter it as the TextFont property in the Inspector. If you also enter zero (0) as the font size, the font size that works best for the platform on which the app is running is used. Because of differences in screen resolutions, different font sizes are often required for each operating system platform. This feature enables you to use different font sizes on different platforms without having to create separate windows for each platform. Use the Inspector to set the font name to “System” and the font size to zero (0).
If the system software supports both a large and small System font, you can also specify the "SmallSystem" font as the font name. This option selects the small system font on the user's computer, if there is one. If there is no small system font, the System font is used.
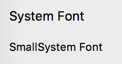
On Mac, both System font and the Small System font are supported.
Available fonts
You may want to use fonts other than the System font. In this case you will need to determine if a particular font is installed on the user's computer. There are two global functions, System.FontCount and Font, that make determining available fonts easy.
The following function, when passed a font name, will return True or False to inform you if the font is installed:
Function FontAvailable(FontName As String) As Boolean
Var i As Integer
For i = 0 To System.FontCount - 1
If System.FontAt(i) = FontName Then
Return True
End If
Next
Return False
End Function
macOS fonts
With Mac apps, not all fonts can appear as Bold, Italic or Underlined even if those properties are selected. Because of the way Mac draws fonts, Bold, Italic and Underline are only displayed for fonts that include it as part of the font definition. These settings cannot be applied to just any font as they can be on other platforms. Instead, on Mac Xojo has to look up the specific font to use based on what you set for font properties.
If you are not seeing the font setting you expect, be sure to verify that the font itself supports it, which you can do using TextEdit or the Font Book app.
On newer version of MacOS (starting with Sierra) your apps may perform slower if you are relying on Xojo to do font lookups. Here is an example of what happens behind the scenes.
Say you want "Arial" in Bold to draw on a Graphics surface of a Canvas with code like this:
g.FontName = "Arial"
g.Bold = True
Xojo takes the font family name (Arial) and the fact that you enabled the Bold property and finds the correct font variant in the "ArialMT" font, which is has this PostScript name: "Arial-BoldMT". It then uses that font to do the actual drawing. You can look up these names yourself using the Mac Font Book app.
If this causes performance issues in your app, you can avoid the lookup by directly specifying the PostScript font name (and not using the Bold or Italic properties). For example instead of the code above, you can do this:
g.FontName = "Arial-BoldMT"
Web fonts
For web apps, you use the control's Style property to set the font or do it through the Inspector.
You can add any of Google's web fonts to your web app as well.
iOS fonts
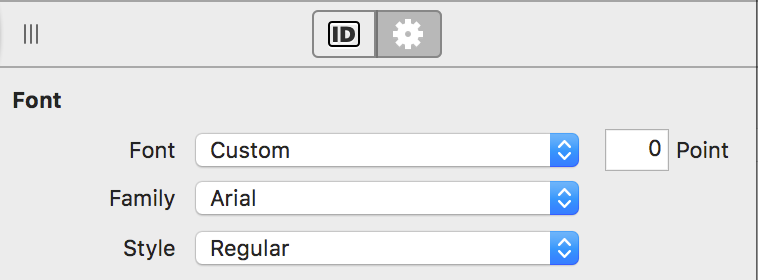
For iOS apps, you use the Font class to specify font settings for display. It has a constructor that takes a parameter for the font's postscript name and point size. For example, this code can set a button text to use Arial at 18 point:
Var courier As New Font("ArialMT", 18)
Button1.CaptionFont = courier
To use the system font, you can use the shared methods like this:
Label1.TextFont = Font.SystemFont(0)
You can also set fonts for the Text Field and Text Area controls using the advanced tab of the Inspector.
See also
Font class; System.FontAt, System.FontCount methods; Changing the Appearance of Web Controls, Creating Styled Text topics