Understanding file types
Your desktop apps may need to prompt users to select or save files. You use File Types to identify the types of files that can be selected or the types of files that are saved, which determines how the OS and other apps can use them.
File Types are created in File Type Groups using the File Type Group Editor. The File Type Group Editor lets you create common file types (file such as text, PNG, JPG, etc.) or custom file types (which are owned by your app).
Using common file types
A common file type is a standard file type that your app uses, but does not own. This may be file types such as png or standard text files. Your app may be able open and even create these type of files, but it is not the owner of the file.
To add a common file type to a File Type Group, choose the Add Common File Type button on the command bar for the File Type Group and choose the one you want. This populates a file type with the necessary information which you should not need to change.
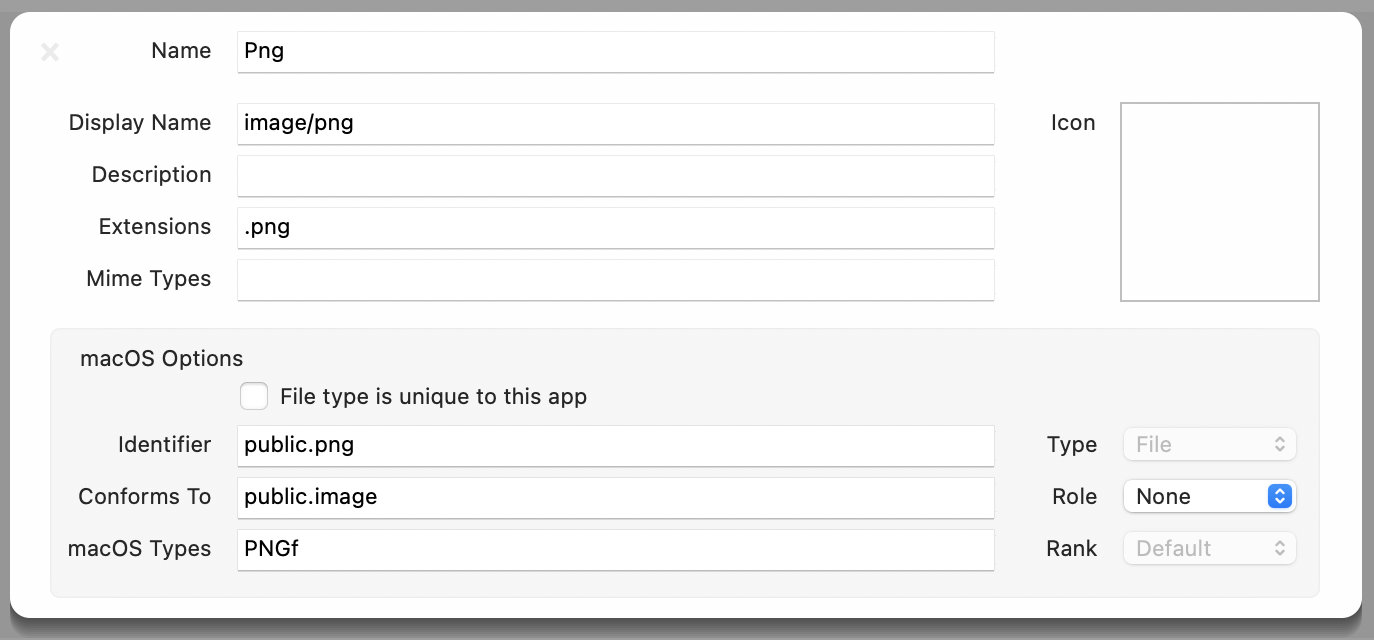
After adding the PNG common file type you can now you can refer to the file type to prompt the user to open a PNG file. This code prompts the user for a file and then assigns it to a Picture property that is displayed in a Canvas:
Var pngFile As FolderItem
pngFile = FolderItem.ShowOpenFileDialog(ImageFileTypeSet.Png)
If pngFile <> Nil Then
PNGImage = Picture.Open(pngFile)
ImageCanvas.Refresh
End If
You can also create your own PNG files. This code saves a PNG file containing a red circle:
Var redCircle As New Picture(100, 100)
redCircle.Graphics.DrawingColor = &cff0000
redCircle.Graphics.FillOval(0, 0, 100, 100)
Var savePNG As FolderItem
savePNG = FolderItem.ShowSaveFileDialog(ImageFileTypeSet.Png, "RedCircle.png")
If savePNG <> Nil Then
redCircle.Save(savePNG, Picture.SaveAsPNG)
End If
Because this is definite as a PNG, the default system icon for PNG is used and displayed when viewing the PNG in the system file viewer.
Using custom file types
A custom file type is a file type where you specify all its properties. For standard file types, you should use a common file type rather than creating your own custom file type. Often you will create a custom file type for file types that your app owns. These are files that your app creates and ones that your app can also open and edit.
Once you have specified a custom File Type, files saved by your app for that type will display the custom icon you specified. Your app will also be launched when the user opens the file (usually be double-clicking it), but the user can change that behavior in the OS itself.
This sample File Type (in a File Type Group called XojoTextFileTypeGroup) is used in the code below:
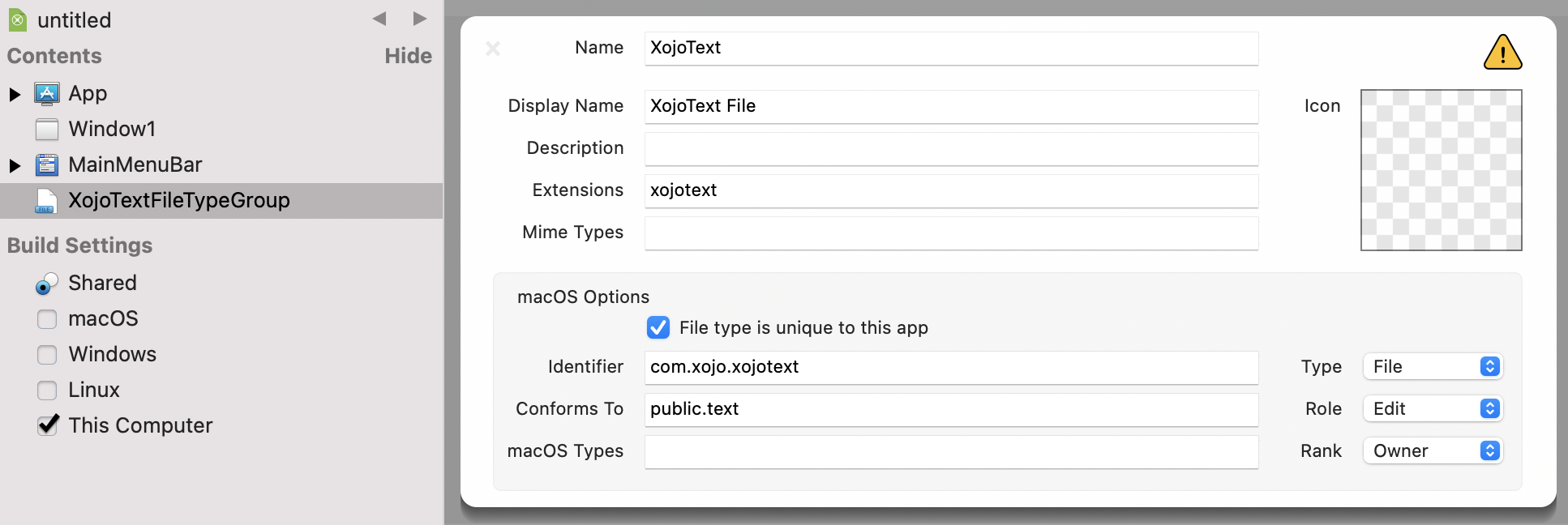
The Identifier, Conforms To, Mime Types and macOS Types fields are only used by macOS.
Saving files
Now that you have the file type specified properly, you can use it to save files of that type. When you prompt the user for the file to save, include the file type from above. This code is in the Action event handler of a Save button on a Window:
Var f As FolderItem
Var output As TextOutputStream
f = FolderItem.ShowSaveFileDialog(XojoTextFileTypeGroup.XojoText, "SampleFile.xojotext")
If f <> Nil then
output = TextOutputStream.Create(f)
output.Write(EditArea.Text)
output.Close
End if
You can also use the SaveAsDialog class for more control of the user file dialog.
On Mac, the saved file will use the icon from the file type, although it's possible the icon does not appear immediately for the associated file. The Finder does not always have time to inspect the app for file types when you are running directly from the IDE. You can always try leaving the debug app running for longer or try building your app and then navigating to its folder. Refer to Troubleshooting for more information.
On Windows, your app installer needs to create Registry entries to set an associated file icon and allow opening of documents to automatically launch the app. For more information on this refer to the sample Inno Setup Scripts for 32-bit and 64-bit apps.
Opening files
When you prompt the user for a file to open, you can also include the file type so that the user can only choose your app's files. This method loads the text from the file and displays it in a Text Field:
Sub LoadFile(f As FolderItem = Nil)
Var file As FolderItem = f
Var input As TextInputStream
If file Is Nil Then
// Prompt user for file to open
file = FolderItem.ShowOpenFileDialog(XojoTextFileTypeGroup.XojoText)
End If
If file <> Nil Then
input = TextInputStream.Open(file)
EditArea.Text = input.ReadAll
input.Close
End If
End Sub
It is called from the Action event handler of an Open button on the Window:
LoadFile
You can also use the OpenDialog class for more control of the user file dialog.
If you need to specify multiple file types, you can just concatenate them like this:
MyGroup.Type1 + MyGroup.Type2
Lastly, when the user double-clicks on one of your app's files 1 , your app will be launched and provided a FolderItem to the file. You'll get this information in the App.OpenDocument event handler, where you can process the file. You will need to have a built app for the OS to launch in order to test this functionality. This code creates a new window and loads the text file into it by calling the method shown above:
Var win As New DocWindow
win.LoadFile(item)
win.Show
The OpenDocument method is also called when the file is dragged onto the app icon (which launches the app) or onto the Dock icon (on macOS).
1 On Windows, your app installer needs to create Registry entries to set an associated file icon and allow opening of documents to automatically launch the app. For more information on this refer to
Creating an Installer with the Inno Setup Script (64-bit apps) and Creating an Installer with the Inno Setup Script (32-bit apps). Also watch the Windows Installers video.
Referencing file types
In addition to the direct ways to refer to a specific file type as shown in the examples above, there are also ways you can also refer to multiple file types. Suppose you create a File Type Set called ImageTypes in which you specify all of the valid image types that your application can open. You can specify the entire list of image types with a line such as:
Var f As FolderItem
f = FolderItem.ShowOpenFileDialog(ImageTypes.All)
If you need to add or remove image file types, your can simply modify the File Type Group and this line of code will automatically refer to the new members of the group.
If you have just a single file type, you can refer to its name directly:
Var f As FolderItem
f = FolderItem.ShowOpenFileDialog(ImageTypes.PNG)
If your File Type Group has multiple file types, you can concatenate them:
Var f As FolderItem
f = FolderItem.ShowOpenFileDialog(ImageTypes.JPEG + ImageTypes.PNG)
Troubleshooting
On macOS, Apple provides some tips to help troubleshoot File Type (UTI) settings:
In particular, some useful commands are:
Command |
Description |
---|---|
mdimport -d1 <file> |
Tells you what UTI it thinks this file conforms to. |
mdimport -d2 <file> |
Tells you what meta data is imported for this file. Look for kMDItemContentTypeTree to see the hierarchy it thinks this file conforms to. |
For anyone with many versions of Xojo installed there will be lots of entries as some will have been imported by the old Spotlight Importer and other things. To test, it is often easier to create a new user account that has never had Xojo installed before using these commands.
Launch services
Per Apple, apps need to be registered in order for them to be known to macOS so that their file type settings work. This is normally taken care of automatically in these situations:
A built-in background tool, run whenever the system is booted or a new user logs in, automatically searches the Applications folders in the system, network, local, and user domains and registers any new applications it finds there.
The Finder automatically registers all apps as it becomes aware of them, such as when they are dragged onto the user's disk or when the user navigates to a folder containing them.
When the user attempts to open a document for which no preferred app can be found in the Launch Services database, the Finder presents a dialog asking the user to select an app with which to open the document. It then registers that app before launching it.
For more information refer to the official Apple Launch Services Concepts document.
Linux notes
Create the MIME type:
sudo -H gedit /etc/mime.types and add the line application/x-xojotext xojotext
Add the icon (which must be called application-x-xojotext.svg):
sudo cp PathToIcon/application-x-xojotext.svg /usr/share/icons/gnome/scalable/mimetypes
(don't forget to replace PathToIcon with the actual path to your icon)